Exception handling in Struts 2
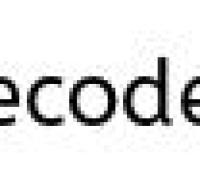
![]() |
|
The Struts 2 framework provides some easy way to handle any uncaught exceptions. It works on exception interceptor which is part of default-stack in struts-default.xml file.
There are two ways to handle uncaught exceptions in Struts2:
- Global exception handling: specifies exception mappings which apply to all action classes in a Struts2.
- Exception handling per action: specifies exception mappings which apply to a specific action class.
Both methods require adding exception mappings in struts.xml configuration file.
** UPDATE: Struts 2 Complete tutorial now available here.
Global Exception Handling :
Add the following code snippet just after the <package> element in struts.xml file:
<global-results> <result name="null">/jsp/NullPointer.jsp</result> <result name="globalException">/jsp/GlobalExceptioon.jsp</result> </global-results> <global-exception-mappings> <exception-mapping result="null" exception="java.lang.NullPointerException"/> <exception-mapping result="globalException" exception="java.lang.Exception"/> </global-exception-mappings>
<global-exception-mappings> – specifies a set of
<global-results> – element defines global view names and its mapping page.
Here in global-exception-mapping the exception of type java.lang.NullPointerException is mapped to the view name “null”. So when any uncaught exception of type java.lang.NullPointerException or its sub types is thrown, Struts will redirect users to the view page mapped with the name “null” and in
Exception Handling Per Action
If you need to handle an exception in a specific way for a certain action you can use the exception-mapping node within the action node.
// Here an action configuration adds an addition exception handler. <action name="Addition" class="simplecode.action.Addition"> <exception-mapping result="error" exception="java.lang.NumberFormatException" /> <result name="success">/jsp/Success.jsp</result> <result name="error">/jsp/Error.jsp</result> </action>
Here If NumberFormatException occurs in Addition action then Error.jsp will be executed else framework searches in the global exception list and execute the respective page using global-result type.
In the Error page we can access information of the exception as follows:
1. Exception name: <s:property value=”exception”/>
2. Exception stack trace: <s:property value=”exceptionStack”/>
3. Exception in the value stack: <s:property value=”[0].top” />
Implementation – exception handling in Struts 2.
File: Struts.xml
<struts> <package name="default" extends="struts-default"> <global-results> <result name="null">/jsp/NullPointer.jsp</result> <result name="globalException">/jsp/GlobalExceptioon.jsp</result> </global-results> // Here the results are expected to be global results. <global-exception-mappings> <exception-mapping result="null" exception="java.lang.NullPointerException"/> <exception-mapping result="globalException" exception="java.lang.Exception"/> </global-exception-mappings> // Here an action configuration adds an addition exception handler. <action name="Addition" class="simplecode.action.Addition"> <exception-mapping result="error" exception="java.lang.NumberFormatException"/> <result name="success">/jsp/Success.jsp</result> <result name="error">/jsp/Error.jsp</result> </action> <action name="Action1" class="simplecode.action.Action1"> <result name="success">/jsp/Success1.jsp</result> </action> <action name="Action2" class="simplecode.action.Action2"> <result name="success">/jsp/Success1.jsp</result> </action> </package> </struts>
Action class
File: Addition.java
For Exception Handling in Action class – here if both numbers is not parsed in int, it will throw NumberFormatException.
package simplecode.action; import com.opensymphony.xwork2.Action; public class Addition implements Action { private String number1, number2; private String sum = ""; public String getNumber1() { return number1; } public String getNumber2() { return number2; } public void setNumber1(String number1) { this.number1 = number1; } public void setNumber2(String number2) { this.number2 = number2; } public String getSum() { return sum; } public void setSum(String sum) { this.sum = sum; } public String execute() throws Exception { int num1 = Integer.parseInt(number1); int num2 = Integer.parseInt(number2); int sum = num1 + num2; setSum("" + sum); return SUCCESS; } }
File: Action1.java – throw NullPointerException
package simplecode.action; import com.opensymphony.xwork2.Action; public class Action1 implements Action { public String execute() throws Exception { String string = null; System.out.println(string.charAt(7)); return SUCCESS; } }
File: Action2.java – throw NumberFormatException.
package simplecode.action; import com.opensymphony.xwork2.Action; public class Action2 implements Action { public String execute() throws Exception { int num = Integer.parseInt("7b"); return SUCCESS; } }
Jsp Pages
File: index.jsp
<%@ taglib uri="/struts-tags" prefix="s"%> <html> <body> <s:form action="Addition"> <s:textfield label="Number 1" name="number1" /> <s:textfield label="Number 2" name="number2" /> <s:submit label="Add" /> </s:form> <hr> Null Pointer Exception example - <a href="<s:url action="Action1"/>">ActionLink1</a> <br> <br> Global Exception - <a href="<s:url action="Action2"/>">ActionLink2</a> <br> </body> </html>
File : Error.jsp
<%@ taglib uri="/struts-tags" prefix="s"%> <html> <body> <h2>Unexpected error occurred (Exception per Action page)</h2> <p> <font color="red">Please contact your system administrator</font> </p> <h3>Error Message</h3> <br> Exception object transferred to value stack <s:property value="[0].top" /> <br> <s:property value="%{exception}" /> <hr /> <h3>Stack Trace</h3> <s:property value="%{exceptionStack}" /> </body> </html>
File: NullPointer.jsp
<%@taglib uri="/struts-tags" prefix="s"%> <html> <body> <h2>Unexpected error occurred (Global - Null Pointer exception)</h2> <p> <font color="red">Please contact your system administrator</font> </p> <h3>Error Message</h3> Exception object transferred to value stack <s:property value="[0].top" /> <br> <s:property value="%{exception}" /> </body> </html>
File: GlobalException.jsp
<%@taglib uri="/struts-tags" prefix="s"%> <html> <head> <title>Global - NumberFormatException</title> </head> <body> <h2>Global NumberFormatException occurred</h2> <p> <font color="red">Please contact your system administrator</font> </p> <h3>Error Message</h3> <br> Exception object transferred to value stack <s:property value="[0].top" /> <br> <s:property value="%{exception}" /> </body> </html>
Demo
http://localhost:8089/Struts2Exception/
On giving the input as above the result will be as shown.
Global exception- On clicking ActionLink1 and ActionLink2 the following global exception page will be rendered.