Struts 2 <s:password> example
In Struts 2 , you can use the <s:password> to create a HTML password field. For example, you can declare the “s:password” with a key attribute or label and name attribute.
<s:password key="password" /> //or <s:textfield label="Password" name="password" />
In Struts 2, the “name” will map to the JavaBean property automatically. In this case, on form submit, the textbox value with “name=’password’” will call the corresponds Action’s setPassword(String xx) to set the value
Struts 2 <s:password> example
A page with ‘password’ and ‘confirm password’ fields, and do the validation to make sure the ‘confirm password’ is match with the ‘password’.
1. Properties file
Two properties files to store the message.
project.properties
project.username = Username project.password= Password project.submit = Login
LoginAction.properties
username.required = Username Cannot be blank password.required = Password Cannot be blank
2. Action
LoginAction.java
package com.simplecode.action; import com.opensymphony.xwork2.ActionSupport; public class LoginAction extends ActionSupport { private static final long serialVersionUID = 1L; private String userName; private String password; public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String execute() { return SUCCESS; } public void validate() { if (userName.equals("")) { addFieldError("userName", getText("username.required")); } if (password.equals("")) { addFieldError("password", getText("password.required")); } } }
3. View page
Result page with Struts 2 “s:password” tag to create a HTML password field.
login.jsp
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <%@taglib uri="/struts-tags" prefix="s"%> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Login</title> </head> <body> <h3>Struts 2 < s:password > Example</h3> <s:form action="welcome"> <s:textfield name="userName" key="project.username" /> <s:password name="password" key="project.password" /> <s:submit key="project.submit" name="submit" /> </s:form> </body> </html>
success.jsp
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>Welcome Page</title> </head> <body> <h3>Struts 2 < s:password > Textbox Examplee</h3> <h4> Username : <s:property value="userName" /><br/> Password : <s:property value = "password"/> </h4> </body> </html>
4. struts.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <constant name="struts.custom.i18n.resources" value="project" /> <package name="default" extends="struts-default" namespace="/jsp"> <action name="Login"> <result>/jsp/login.jsp</result> </action> <action name="welcome" class="com.simplecode.action.LoginAction"> <result name="success">/jsp/success.jsp</result> <result name="input">/jsp/login.jsp</result> </action> </package> </struts>
5. Demo
http://localhost:8089/Struts2_password/
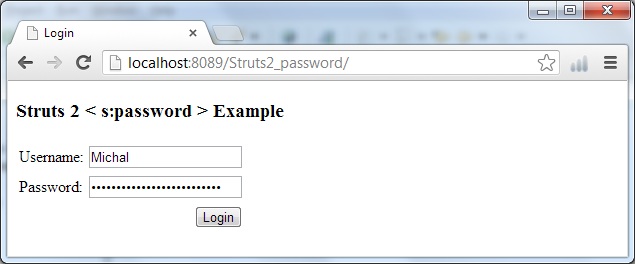
Reference
- Struts 2 password documentation
I simply want to mention I am beginner to blogging and absolutely liked this website. More than likely I’m want to bookmark your website . You surely come with exceptional stories. Many thanks for sharing your website.