Pagination in Struts 2 using jQuery jTable plugin
In the previous article “Setting up JQuery jTable plugin in Struts 2″ I have explained how to setup jTable plugin in struts 2 and in the article “Ajax based curd operation in Struts 2 using JQuery jTables plugin” I have implemented Ajax based curd operation using JTable plugin.
This is the third article on jQuery jTable plugin in Struts 2 that describes on how to implement pagination feature to do server side paging in struts 2 using the JQuery jTable plugin and it will not explain how to setup jTable plugin in struts 2 here. So If you have not read the previous articles “Setting up JQuery jTable plugin in Struts 2″ and “Ajax based curd operation in Struts 2 using JQuery jTables plugin”, I will recommend that you read that article first because first one explains how you can integrate the JTable plug-in with a Struts 2 application and in second article explains on how to implement ajax based curd operation using jTable plugin. This article will assume that the code for the integration of the jQuery JTable plug-in is implemented, and only the code required for implementing pagination in Struts 2 using jTable will be explained here.
Setup
As described above, the prerequisite for this code is that you integrate the jQuery jTable plugin into the Struts 2. You can find detailed instructions here, at JQuery jTable plugin in Struts 2, so now I am going to take the sample application I created for explaining AJAX based crud operations in jTable plugin and continue to implement paging for that application.
1. Download sample application from here and import the project in eclipse
2. Follow the steps in this article here to create table in database.
Steps to enable Paging:
From the browser perspective: jTable
To enable paging, paging option must set to true. You can also set pageSize option (default value is 10) in jQuery Script code.
$('#StudentTableContainer').jtable({ //... paging: true, //Set paging enabled pageSize: 3, //Set page size actions: { //... }, fields: { //... } });
Note: pageSize sets the initial number of records to be displayed per page.
Modified Jsp page
<!DOCTYPE html> <html> <head> <title>jTable Pagination in Java Web Applications</title> <!-- Include one of jTable styles. --> <link href="css/metro/blue/jtable.css" rel="stylesheet" type="text/css" /> <link href="css/jquery-ui-1.10.3.custom.css" rel="stylesheet" type="text/css" /> <!-- Include jTable script file. --> <script src="js/jquery-1.8.2.js" type="text/javascript"></script> <script src="js/jquery-ui-1.10.3.custom.js" type="text/javascript"></script> <script src="js/jquery.jtable.js" type="text/javascript"></script> <script type="text/javascript"> $(document).ready(function() { $('#StudentTableContainer').jtable({ title : 'Students List', paging : true, //Enable paging pageSize : 3, //Set page size (default: 10) actions : { listAction : 'listAction', createAction : 'createAction', updateAction : 'updateAction', deleteAction : 'deleteAction' }, fields : { studentId : { title : 'Student Id', width : '30%', key : true, list : true, edit : false, create : true }, name : { title : 'Name', width : '30%', edit : true }, department : { title : 'Department', width : '30%', edit : true }, emailId : { title : 'Email', width : '20%', edit : true } } }); $('#StudentTableContainer').jtable('load'); }); </script> </head> <body> <div style="text-align: center;"> <h3>jTable Pagination in Java Web Applications</h3> <div id="StudentTableContainer"></div> </div> </body> </html>
From the server’s perspective: Servlet
If paging is enabled, jTable sends two query string parameters to the server on listAction AJAX call:
• jtStartIndex: Start index of records for current page.
• jtPageSize: Count of maximum expected records.
And it expects additional information from server:
• TotalRecordCount: Total count of records.
In our previous example the url specified in the ‘listAction‘ option has business logic to fetch all records from database. Now in order to handle pagination this ‘listAction’ option should fetch only a part of records for each page, So handle this there are two changes that has to be done in the server side .
1. Modify oracle query to fetch only a subset of records based on the jtStartIndex and jtPageSize.
Since these values are sent along with the request as string parameters by jTable so add following member variable in struts 2 action class and create getters and setters for the same
// Holds Start Page Index private int jtStartIndex; // Hold records to be displayed per Page private int jtPageSize;
2. As mentioned above, jTable need TotalRecordCount to be present in the json response, For which add a member variable totalRecordCount in struts 2 action class and create getters and setters for the same.
Now replace the list method in action class with the below code,
public String list() { try { // Fetch Data from Student Table records = dao.getAllStudents(jtStartIndex, jtPageSize); // Get Total Record Count for Pagination totalRecordCount = dao.getStudentCount(); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); } return Action.SUCCESS; }
Changes made at Dao class
Add below two methods in ‘CrudDao.java’ file
1. Method to get the count of total number of records in the result set.
public int getStudentCount() { int count=0; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery("SELECT COUNT(*) AS COUNT FROM STUDENT"); while (rs.next()) { count=rs.getInt("COUNT"); } } catch (SQLException e) { System.err.println(e.getMessage()); } return count; }
2. In order to return only a subset of records according to the page offset (jtStartIndex and jtPageSize), oracle query should be modified as follows,
In case of Oracle database:
“SELECT * from (Select M.*, Rownum R from STUDENT M) where r > ” + < jtStartIndex> +” and r <= "+< jtStartIndex + jtPageSize >;
In case of MySql database:
select * from STUDENT limit <jtStartIndex>,<jtPageSize>
Now modify getAllUsers in CurdDao.java using below code
public List<Student> getAllStudents(int startPageIndex, int recordsPerPage) { List<Student> students = new ArrayList<Student>(); int range = startPageIndex+recordsPerPage; String query="SELECT * from (Select M.*, Rownum R From STUDENT M) where r > " + startPageIndex +" and r <= "+range; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); while (rs.next()) { Student student = new Student(); student.setStudentId(rs.getInt("STUDENTID")); student.setName(rs.getString("NAME")); student.setDepartment(rs.getString("DEPARTMENT")); student.setEmailId(rs.getString("EMAIL")); students.add(student); } } catch (SQLException e) { System.err.println(e.getMessage()); } return students; }
This function returns only a subset of records according to the page offset (jtStartIndex and jtPageSize).
Now on running the application, with the above changes, the final demo looks as shown below:
![]() |
|
Read More
CRUD Operations in Struts 2 using jTable jQuery plugin via Ajax
In the previous article “Setting up jQuery jTable plugin with Struts 2 framework” I have explained about how to setup jTable plugin in struts 2 application. This article describes on how to implement “Ajax based curd operation in Struts 2 using the JQuery jTable plugin, If you have not read the previous articles “Setting up jQuery jTable plugin with Struts 2 framework” I will recommend that you read that article first because it explains what jTable plugin is and how you can integrate it in Struts 2 application.
Steps done to set up our application for jTable
Libraries required for the setup,
- jQuery
- jQuery UI
- jTable
- struts2-json-plugin-2.x.x.jar
- Commonly required Struts 2 jars
Create a dynamic project in eclipse and setup above required libraries as explained here. The final project structure of this looks as below.
Setup from the browser perspective: jTable
JSP
<html> <head> <title>jTable in Struts 2</title> <!-- jTable metro styles. --> <link href="css/metro/blue/jtable.css" rel="stylesheet" type="text/css" /> <link href="css/jquery-ui-1.10.3.custom.css" rel="stylesheet" type="text/css" /> <!-- jTable script file. --> <script src="js/jquery-1.8.2.js" type="text/javascript"></script> <script src="js/jquery-ui-1.10.3.custom.js" type="text/javascript"></script> <script src="js/jquery.jtable.js" type="text/javascript"></script> <!-- User defined Jtable js file --> <script src="js/userDefinedJtable.js" type="text/javascript"></script> </head> <body> <div style="text-align: center;"> <h3>AJAX based CRUD operation in Struts 2 using jTable plugin</h3> <div id="StudentTableContainer"></div> </div> </body> </html>
JS file for implementing CRUD
$(document).ready(function() { $('#StudentTableContainer').jtable({ title : 'Students List', actions : { listAction : 'listAction', createAction : 'createAction', updateAction : 'updateAction', deleteAction : 'deleteAction' }, fields : { studentId : { title : 'Student Id', width : '30%', key : true, list : true, edit : false, create : true }, name : { title : 'Name', width : '30%', edit : true }, department : { title : 'Department', width : '30%', edit : true }, emailId : { title : 'Email', width : '20%', edit : true } } }); $('#StudentTableContainer').jtable('load'); });
I have explained the working of above jTable js file in my previous article “Setting up JQuery jTable plugin in Struts 2″, hence I’m not going to explain it again.
Now create a student table in Oracle database using the query below. On this table we are going to perform CRUD operation via ajax
create table Student(studentid int,name varchar(50),department varchar(50),email varchar(50));
CurdDao
Create a class that performs CRUD operation in database
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.List; import com.jdbc.DataAccessObject; import com.model.Student; public class CrudDao { private Connection dbConnection; private PreparedStatement pStmt; public CrudDao() { dbConnection = DataAccessObject.getConnection(); } public void addStudent(Student student) { String insertQuery = "INSERT INTO STUDENT(STUDENTID, NAME, " + "DEPARTMENT, EMAIL) VALUES (?,?,?,?)"; try { pStmt = dbConnection.prepareStatement(insertQuery); pStmt.setInt(1, student.getStudentId()); pStmt.setString(2, student.getName()); pStmt.setString(3, student.getDepartment()); pStmt.setString(4, student.getEmailId()); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public void deleteStudent(int userId) { String deleteQuery = "DELETE FROM STUDENT WHERE STUDENTID = ?"; try { pStmt = dbConnection.prepareStatement(deleteQuery); pStmt.setInt(1, userId); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public void updateStudent(Student student) { String updateQuery = "UPDATE STUDENT SET NAME = ?, " + "DEPARTMENT = ?, EMAIL = ? WHERE STUDENTID = ?"; try { pStmt = dbConnection.prepareStatement(updateQuery); pStmt.setString(1, student.getName()); pStmt.setString(2, student.getDepartment()); pStmt.setString(3, student.getEmailId()); pStmt.setInt(4, student.getStudentId()); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public List<Student> getAllStudents() { List<Student> students = new ArrayList<Student>(); String query = "SELECT * FROM STUDENT ORDER BY STUDENTID"; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); while (rs.next()) { Student student = new Student(); student.setStudentId(rs.getInt("STUDENTID")); student.setName(rs.getString("NAME")); student.setDepartment(rs.getString("DEPARTMENT")); student.setEmailId(rs.getString("EMAIL")); students.add(student); } } catch (SQLException e) { System.err.println(e.getMessage()); } return students; } }
I hope the above code is self explanatory
Setup from the server’s perspective: Servlet
In Struts 2 Action class, I have defined 4 method- create, read, update and delete to perform CRUD operations. Since jTable accepts data only in Json format, so we are converting this List (Java Object) to Json(Javascript object Notation) format using struts2-json-plugin.jar.
**Update: In the article AJAX implementation in Struts 2 using JQuery and JSON I have explained in detail about how to use struts2-json-plugin.jar clearly, So if you are not aware of how struts2-json-plugin works, then please go thorough the above mentioned link.
Action class
package com.action; import java.io.IOException; import java.util.List; import com.dao.CrudDao; import com.model.Student; import com.opensymphony.xwork2.Action; public class JtableAction { private CrudDao dao = new CrudDao(); private List<Student> records; private String result; private String message; private Student record; private int studentId; private String name; private String department; private String emailId; public String list() { try { // Fetch Data from Student Table records = dao.getAllStudents(); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String create() throws IOException { record = new Student(); record.setStudentId(studentId); record.setName(name); record.setDepartment(department); record.setEmailId(emailId); try { // Create new record dao.addStudent(record); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String update() throws IOException { Student student = new Student(); student.setStudentId(studentId); student.setName(name); student.setDepartment(department); student.setEmailId(emailId); try { // Update existing record dao.updateStudent(student); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String delete() throws IOException { // Delete record try { dao.deleteStudent(studentId); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public int getStudentId() { return studentId; } public String getName() { return name; } public String getDepartment() { return department; } public String getEmailId() { return emailId; } public void setStudentId(int studentId) { this.studentId = studentId; } public void setName(String name) { this.name = name; } public void setDepartment(String department) { this.department = department; } public void setEmailId(String emailId) { this.emailId = emailId; } public Student getRecord() { return record; } public void setRecord(Student record) { this.record = record; } public List<Student> getRecords() { return records; } public String getResult() { return result; } public String getMessage() { return message; } public void setRecords(List<Student> records) { this.records = records; } public void setResult(String result) { this.result = result; } public void setMessage(String message) { this.message = message; } }
If you read my article on CRUD Operations in Java Web Applications using jTable jQuery plugin via Ajax then you might have noted once difference here, i.e. I have not created any request or response object in action class to get the student parameters, because those parameter from jsp file auto bounded to my struts 2 action, this is done via struts2-jquery-plugin. One only requirement for this parameter to be passed from jsp is, you have create the member variable for those parameter in action class along with getters and setters as in above file.
Do read:
I have explained in detail about difference response generated for create, read, update and delete operation in the article CRUD Operations in Java Web Applications using jTable jQuery plugin via Ajax, So please refer to this article mentioned above, if you are not aware of the different response created for CRUD operation in Jtable plugin.
Jtable Issue related to Struts 2
As mentioned in my previous article , the property names of jTable plugin are case sensitive. Only “Result”, “Records” and “Message” will work. In struts 2 the “json response” generated is in lower case["result", "records" and "message"], hence I edited the jtable.js to replace Result to result, Records to records and Message to message then it worked.
**Note: Along with the above keyword replace TotalRecordCount to totalRecordCount, since this parameter will be used to display pagination count (Which I will implement in upcoming tutorial)
Model class
Create Model class used in the controller, which will have getters and setters for fields specified in jTable script.
package com.model; public class Student { private int studentId; private String name; private String department; private String emailId; public int getStudentId() { return studentId; } public String getName() { return name; } public String getDepartment() { return department; } public String getEmailId() { return emailId; } public void setStudentId(int studentId) { this.studentId = studentId; } public void setName(String name) { this.name = name; } public void setDepartment(String department) { this.department = department; } public void setEmailId(String emailId) { this.emailId = emailId; } }
DAO Class
Create utility class which connect to database Using Oracle JDBC driver
package com.jdbc; import java.sql.Connection; import java.sql.DriverManager; public class DataAccessObject { private static Connection connection = null; public static Connection getConnection() { if (connection != null) return connection; else { // database URL String dbUrl = "jdbc:oracle:thin:@localhost:1521:XE"; try { Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the database connection = DriverManager.getConnection(dbUrl, "system", "admin"); } catch (Exception e) { e.printStackTrace(); } return connection; } } }
Struts.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" extends="json-default"> <action name="*Action" class="com.action.JtableAction" method="{1}"> <result type="json">/jTable.jsp</result> </action> <action name="getJSONResult" class="com.action.JtableAction" method="list"> <result type="json" /> </action> </package> </struts>
web.xml
<filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list>
Demo
On running the application
On clicking ‘Add new record’
Now the new record will be added with fade out animation
On clicking edit button
On clicking delete button
In the next article Pagination in Struts 2 using jQuery jTable plugin I have implemented paging feature in the CRUD example.
![]() |
|
Reference
Read MoreAutocomplete in Struts 2 using Jquery and JSON via Ajax
I have already written a detailed post on Autocompleter Textbox & dropdown in Struts 2 using struts2-dojo-plugin.jar. In this post, I am going to describe how to implement Ajax based autocomplete in Struts 2 web application using jQuery plugin. jQuery Autcomplete is part of the jQuery UI library which allows converting a normal textbox into an autocompleter textbox by providing a data source for the autocompleter values.
Here when user types a character in text box, jQuery will fire an ajax request using autocomplete plugin to Struts 2 action class, which in turn call the dao class which connects to the database and returns the required data back as an array list, this list should be returned in json format to the success function of ajax call. So to handle this you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute (which has getter and setter) into a JSON object. This guide will teach you on how to implementation AJAX in Struts 2 using JQuery and JSON
Library
struts2-json-plugin-2.x.x.jar
ojdbc14.jar
jquery-1.10.2.js
jquery-ui.js
jquery-ui.css
Now create a dynamic web project in eclipse and add the above jars in classpath of the project and the project structure should look like shown below.
Project Structure
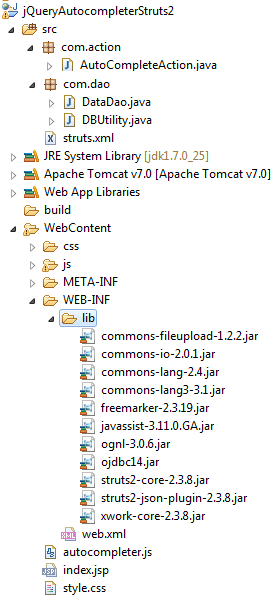
Jsp page
We are done with the setup. Now create a new jsp file under WebContent folder to display the data fetched from database into autocomplete textbox. Now to implement this page with Autocompleter feature and make sure that you referred the jQuery core and jQueryUI libraries.
<%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html> <html> <head> <meta charset="ISO-8859-1"> <title>Autocomplete in Struts 2 using Jquery and JSON</title> <script src="js/jquery-1.10.2.js"></script> <script src="js/jquery-ui.js"></script> <script src="autocompleter.js"></script> <link rel="stylesheet" href="css/jquery-ui.css"> <link rel="stylesheet" href="style.css"> </head> <body> <div class="header"> <h3>Autocomplete in Struts 2 using Jquery and JSON</h3> </div> <br /> <br /> <div class="search-container"> <div class="ui-widget"> <s:textfield id="search" name="search" /> </div> </div> </body> </html>
Js file
Here we get data from database via ajax and apply autocompleter
$(document).ready(function() { $(function() { $("#search").autocomplete({ source : function(request, response) { $.ajax({ url : "searchAction", type : "POST", data : { term : request.term }, dataType : "json", success : function(jsonResponse) { response(jsonResponse.list); } }); } }); }); });
When a user types a character in text box ,jQuery will fire an ajax request to the controller, in this case controller is SearchController as mentioned in the above js file.
Recommended reading :
Business class
Next step is to create a class that would fetch data from database.
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; public class DataDao { private Connection connection; public DataDao() throws Exception { connection = DBUtility.getConnection(); } public ArrayList<String> getFrameWork(String frameWork) { ArrayList<String> list = new ArrayList<String>(); PreparedStatement ps = null; String data; try { ps = connection .prepareStatement("SELECT * FROM JAVA_FRAMEWORK WHERE FRAMEWORK LIKE ?"); ps.setString(1, frameWork + "%"); ResultSet rs = ps.executeQuery(); while (rs.next()) { data = rs.getString("FRAMEWORK"); list.add(data); } } catch (Exception e) { System.out.println(e.getMessage()); } return list; } }
Data Access object
Connecting To Database Using JDBC
package com.dao; import java.sql.Connection; import java.sql.DriverManager; public class DBUtility { private static Connection connection = null; public static Connection getConnection() { if (connection != null) return connection; else { // database URL String dbUrl = "jdbc:oracle:thin:@localhost:1521:XE"; try { Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the database connection = DriverManager.getConnection(dbUrl, "system", "admin"); } catch (Exception e) { e.printStackTrace(); } return connection; } } }
Action class
Now Create the action class to handle Ajax call; in action class you need to create instance variables and its respective getter and setter methods since all the variables which have a setter can be set to the values as which are passed as parameters by jQuery and all the variables that have a getter method can be retrieved in the client javascript code.
package com.action; import java.util.ArrayList; import com.dao.DataDao; import com.opensymphony.xwork2.Action; public class AutoCompleteAction implements Action { // Received via Ajax request private String term; // Returned as responce private ArrayList<String> list; public String execute() { try { System.out.println("Parameter from ajax request : - " + term); DataDao dataDao = new DataDao(); list = dataDao.getFrameWork(term); } catch (Exception e) { System.err.println(e.getMessage()); } return SUCCESS; } public ArrayList<String> getList() { return list; } public void setList(ArrayList<String> list) { this.list = list; } public String getTerm() { return term; } public void setTerm(String term) { this.term = term; } }
As mentioned in the code, the action class will call the business service class which in turn creates the necessary connection and returns the data back as an array list.
Also read :
struts.xml
In struts.xml, create a package that extend json-default and specify the result type of your action class inside this package to be json. This package component is present in struts2-json-plugin-2.x.x.jar
Please read the article on AJAX implementation in Struts 2 using JQuery and JSON to understand about “json-default” package better.
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" extends="json-default"> <action name="searchAction" class="com.action.AutoCompleteAction"> <result type="json">index.jsp</result> </action> </package> </struts>
web.xml
Make sure you have done mapping in web.xml file as given below,
<filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list>
Demo
![]() |
|
If you have any other suggestion on above topic, do let me know via comments. Don’t forget to share this article for comment moderation with your blogger friends. Read More
GridView in Struts2 using jQuery DataTable via Ajax
In this post, I am going to explain on how to use DataTable plugin to display data in Gridview format in Struts 2 web application.
DataTable is a jQuery plugin which adds a lot of functionality to plain HTML tables, such as filtering, paging sorting, changing page length, server side processing etc.
Library
- struts2-json-plugin-x.x.x.jar
- DataTable Plugin
- OpenCSV(Optional – I am using this to deal with a csv file as data source instead of database to keep it simple)
- jQuery UI themes
In this example, I am going to retrieve values from a csv file and display it in html table. For this, I am going to use OpenCSV library which simplifies the work of parsing CSV files. Here the Data table will load the data by making an Ajax call.
Note:
• Refer the article on how to Read / Write CSV file in Java using Opencsv library/ .
Since the response to be generated from the action class is of type JSON, So to handle it you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute (which has getter and setter) into a JSON object. Refer this article here for more detail.
Now create a dynamic web project in eclipse and create two folders named ‘js’ and ‘css’ under WebContent, and add the following javascript files from DataTable to the ‘js’ folder
• jquery.dataTables.js
• jquery.js
Add the following css files from DataTable & jQuery ui to ‘css’ folder.
• demo_page.css
• demo_table_jui.css
• jquery-ui-x.x.x.css
** UPDATE: Struts 2 Complete tutorial now available here.
Download the csv file from which the data is to be read from here and place it under src folder. This css files contains four columns of csv file – company, country, revenue, and year.
Project Structure
Model class
Create a model class that gets and sets the data from the four columns (company, country, revenue, and year) of the csv file.
package com.model; public class RevenueReport { public RevenueReport(String company, String country, String year, String revenue) { this.company = company; this.country = country; this.year = year; this.revenue = revenue; } private String company; private String country; private String year; private String revenue; public String getCountry() { return country; } public String getRevenue() { return revenue; } public String getCompany() { return company; } public String getYear() { return year; } public void setCountry(String country) { this.country = country; } public void setRevenue(String revenue) { this.revenue = revenue; } public void setCompany(String company) { this.company = company; } public void setYear(String year) { this.year = year; } }
Business class
Create a Business Service class that would fetch data from the csv file using model class.
package com.service; import java.io.*; import java.io.InputStreamReader; import java.util.LinkedList; import java.util.List; import au.com.bytecode.opencsv.CSVReader; import com.model.RevenueReport; public class BusinessService { public static List<RevenueReport> getCompanyList() { List<RevenueReport> listOfCompany = new LinkedList<RevenueReport>(); String fileName = "Company_Revenue.csv"; InputStream is = Thread.currentThread().getContextClassLoader() .getResourceAsStream(fileName); BufferedReader br = new BufferedReader(new InputStreamReader(is)); try { CSVReader reader = new CSVReader(br); String[] row = null; while ((row = reader.readNext()) != null) { listOfCompany.add(new RevenueReport(row[0], row[1], row[2], row[3])); } reader.close(); } catch (IOException e) { System.err.println(e.getMessage()); } return listOfCompany; } }
Jsp
Now create the jsp file to display the data fetched from csv file in html table and enhance the table features using DataTable plugin.
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Gridview in Struts2 using jQuery DataTable plugin</title> <link href="css/demo_table_jui.css" rel="stylesheet" /> <link href="css/jquery-ui.css" rel="stylesheet" /> <link href="css/demo_page.css" rel="stylesheet" /> <script src="js/jquery.js"></script> <script src="js/jquery.dataTables.js"></script> <script> $(document).ready(function() { $(".jqueryDataTable").dataTable({ "sPaginationType" : "full_numbers", "bProcessing" : false, "bServerSide" : false, "sAjaxSource" : "dataTablesAction", "bJQueryUI" : true, "aoColumns" : [ { "mData": "company" }, { "mData": "country" }, { "mData": "year" }, { "mData": "revenue" } ] } ); } ); </script> </head> <body id="dt_example"> <div id="container"> <h1>Ajax based Gridview in Struts2 using jQuery DataTable plugin</h1> <div id="demo_jui"> <table class="display jqueryDataTable"> <thead> <tr> <th>Company</th> <th>Country</th> <th>Year</th> <th>Revenue</th> </tr> </thead> <tbody> </tbody> </table> </div> </div> </body> </html>
Action class
In reply to each request for information that DataTables makes to the server, it expects to get a well formed JSON object with the parameter below.
1. aaData- The data in a 2D array.
package com.action; import java.util.List; import com.model.RevenueReport; import com.opensymphony.xwork2.Action; import com.service.BusinessService; public class GridViewAction implements Action { private List<RevenueReport> aaData; public List<RevenueReport> getAaData() { return aaData; } public void setAaData(List<RevenueReport> aaData) { this.aaData = aaData; } public String execute() { aaData = BusinessService.getCompanyList(); return SUCCESS; } }
struts.xml
<struts> <package name="default" extends="json-default"> <action name="dataTablesAction" class="com.action.GridViewAction"> <result type="json">grid.jsp</result> </action> </package> </struts>
Note that I have extended “json-default” package instead of struts-default package and I have set the result type to json, I have explained about the reason for extending “json-default” package in the article AJAX implementation in Struts 2 using JQuery and JSON, please refer the mentioned link if you are not aware of the same.
web.xml
Make sure you have done servlet mapping properly in web.xml file. An example of this is given below,
<web-app> <display-name>Struts2</display-name> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
Demo
![]() |
|
Read More
AJAX implementation in Struts 2 using JQuery and JSON
In this post, we will learn to implement AJAX calls from a JSP page to a Struts 2 Action class using JQuery and update the same JSP page back with the Json response from the Struts 2.
Library required
Since the response to be sent to jQuery is of type JSON, So to handle it you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute which has getter and setter into a JSON object.
Steps done to set up our action for JSON
From the browser perspective: jQuery
jQuery allows you to issue an ajax request and expects a JSON object as a response.
Jsp Page
Now, let us create a JSP page with two drop down lists, one contains values for countries and the other that is going to be populated with values for states based on the value selected in the first drop down list. This is done without a page refresh, by making AJAX calls to the Struts 2 action on first drop down list change event.
Recommended reading :
<%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>AJAX in Struts 2 using JSON and jQuery</title> <script src="js/jquery-1.8.2.js" type="text/javascript"></script> <script> $(document).ready(function() { $('#country').change(function(event) { var country = $("select#country").val(); $.getJSON('ajaxAction', { countryName : country }, function(jsonResponse) { $('#ajaxResponse').text(jsonResponse.dummyMsg); var select = $('#states'); select.find('option').remove(); $.each(jsonResponse.stateMap, function(key, value) { $('<option>').val(key).text(value).appendTo(select); }); }); }); }); </script> </head> <body> <h3>AJAX calls to Struts 2 using JSON and jQuery</h3> <s:select id="country" name="country" list="{'Select Country','India','US'}" label="Select Country" /> <br /> <br /> <s:select id="states" name="states" list="{'Select State'}" label="Select State" /> <br /> <br /> <div id="ajaxResponse"></div> </body> </html>
Note that I have referenced jQuery files in the head section of the jsp which is responsible for the AJAX call made to the struts 2 action and for displaying the response back in the JSP.
Whenever the value is selected in the “country” drop down list, its change event is fired and the ‘getJSON’ function executes ajaxAction (first argument of ‘getJSON’) configured in struts.xml. Since I have explained about the parameters of ‘getJSON’ method in the article here, so I’m not going to explain about it again.
From the server’s perspective: Struts 2
In action class you need to create instance variables and its respective getter and setter methods. Here all the variables that have a setter can be set to the values received as parameters from jQuery and all the variables that have a getter method can be retrieved in the client javascript code.
Also read :
Action class
package com.action; import java.util.LinkedHashMap; import java.util.Map; import com.opensymphony.xwork2.Action; public class AjaxJsonAction implements Action{ private Map<String, String> stateMap = new LinkedHashMap<String, String>(); private String dummyMsg; //Parameter from Jquery private String countryName; public String execute() { if (countryName.equals("India")) { stateMap.put("1", "Kerala"); stateMap.put("2", "Tamil Nadu"); stateMap.put("3", "Jammu Kashmir"); stateMap.put("4", "Assam"); } else if (countryName.equals("US")) { stateMap.put("1", "Georgia"); stateMap.put("2", "Utah"); stateMap.put("3", "Texas"); stateMap.put("4", "New Jersey"); } else if (countryName.equals("Select Country")) { stateMap.put("1", "Select State"); } dummyMsg = "Ajax action Triggered"; return SUCCESS; } public Map<String, String> getStateMap() { return stateMap; } public String getDummyMsg() { return dummyMsg; } public String getCountryName() { return countryName; } public void setStateMap(Map<String, String> stateMap) { this.stateMap = stateMap; } public void setDummyMsg(String dummyMsg) { this.dummyMsg = dummyMsg; } public void setCountryName(String countryName) { this.countryName = countryName; } }
In the above code, we create a maps and populates its value based on the country parameter passed to the action class by the AJAX call made by the JQuery’s getJSON() method.
struts.xml
Since the response needed by jQuery is of type json, so we need to convert this map objects to json strings, for this you need to configure this Action class in struts.xml such that it returns a json object. This configuration is as follows:
Create a package in struts.xml file, which extend json-default and specify the result type of your action class inside this package to be json. The json-default package contains an interceptor and the result type configuration for JSON requests and responses.
<struts> <package name="default" extends="json-default"> <action name="ajaxAction" class="com.action.AjaxJsonAction"> <result type="json">/index.jsp</result> </action> </package> </struts>
Note: json-default package extends struts-default
Web.xml
<display-name>Struts2</display-name> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
Demo
On selecting ‘India’ in the first dropdown list
On selecting ‘US’ in the first dropdown list
![]() |
|
Read More
Struts 2 and Tiles Framework Integration
Apache Tiles is a templating framework used to simplify the development of web application user interfaces. Tiles allow defining page fragments which can be combined into a complete page at runtime. These fragments, or tiles, can be used as reusable templates in order to reduce the duplication of common page elements or even embedded within other tiles. See the snapshot below.
** UPDATE: Struts 2 Complete tutorial now available here.
Advantage of tiles framework
- Code reusability
- Easy to modify
- Easy to remove
Jar Required
Folder structure
web.xml
Provide entry of listener class Struts2TilesListener in the web.xml file.
<listener> <listener-class>org.apache.struts2.tiles.StrutsTilesListener</listener-class> </listener>
Jsp Pages
Now We will define the template for our web application in baseLayout.jsp file. This template will contain different segments of web page (Header, Footer, Menu, Body).
File : baseLayout.jsp
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title><tiles:insertAttribute name="title" ignore="true" /></title> <style> .one { border-style: solid; border-color: #0000ff; border-collapse: collapse; } </style> </head> <body> <table border="1" align="center" class="one" width="80%"> <tr> <td height="30" colspan="2" class="one" width="20%"> <tiles:insertAttribute name="header" /> </td> </tr> <tr> <td height="250" class="one"> <tiles:insertAttribute name="menu" /> </td> <td width="350" class="one"> <tiles:insertAttribute name="body" /> </td> </tr> <tr> <td height="30" colspan="2" class="one"> <tiles:insertAttribute name="footer" /> </td> </tr> </table> </body> </html>
File : header.jsp
<html> <body> <div align="center" style="color: gray; font-weight: bold;"> Struts 2 - Tiles Demo - Header page </div> </body> </html>
File : footer.jsp
<html> <body> <div align="center" style="color: gray; font-weight: bold;"> Footer page © SimpleCodeStuffs.com </div> </body> </html>
File : body.jsp
<html> <body> <p align="center" style="color: gray;font-weight: bold;">Home Page</p> </body> </html>
File : menu.jsp
<%@taglib prefix="s" uri="/struts-tags"%> <html> <body> <s:url action="strutsAction" var="strutsAction" /> <s:url action="springAction" var="springAction" /> <div align="center"> Menu <br /> <br /> <br /> <br /> <s:a href="%{strutsAction}">Struts Tutorial</s:a> <br /> <br /> <s:a href="%{springAction}">Spring Tutorial</s:a> </div> <br> </body> </html>
File : springTutorial.jsp
<html> <body> <p align="center" style="color: gray;font-weight: bold;">Spring Tutorial !!!</p> </body> </html>
File : strutsTutorial.jsp
<html> <body> <p align="center" style="color: gray;font-weight: bold;">Struts Tutorial !!!</p> </body> </html>
File : index.jsp
It triggers homeAction during application start up
<META HTTP-EQUIV="Refresh" CONTENT="0;URL=homeAction">
Action Class
package com.simplecodestuffs.action; public class TilesAction { public String home() { return "home"; } public String struts() { return "struts"; } public String spring() { return "spring"; } }
struts.xml
In struts.xml, inherit the tiles-default package and define all the result type as tiles
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" extends="tiles-default"> <action name="*Action" method="{1}" class="com.simplecodestuffs.action.TilesAction"> <result name="home" type="tiles">home</result> <result name="struts" type="tiles">struts</result> <result name="spring" type="tiles">spring</result> </action> </package> </struts>
tiles.xml
The tiles.xml file must be located inside the WEB-INF directory, in which define all the tiles definitions
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 2.0//EN" "http://tiles.apache.org/dtds/tiles-config_2_0.dtd"> <tiles-definitions> <definition name="baseLayout" template="/baseLayout.jsp"> <put-attribute name="title" value="Template" /> <put-attribute name="header" value="/header.jsp" /> <put-attribute name="menu" value="/menu.jsp" /> <put-attribute name="body" value="/body.jsp" /> <put-attribute name="footer" value="/footer.jsp" /> </definition> <definition name="home" extends="baseLayout"> <put-attribute name="title" value="Home" /> <put-attribute name="body" value="/home.jsp" /> </definition> <definition name="struts" extends="baseLayout"> <put-attribute name="title" value="Struts Tutorial" /> <put-attribute name="body" value="/strutsTutorial.jsp" /> </definition> <definition name="spring" extends="baseLayout"> <put-attribute name="title" value="Spring Tutorial" /> <put-attribute name="body" value="/springTutorial.jsp" /> </definition> </tiles-definitions>
Demo
Home Page with Tiles
Struts Page with Tiles
Spring Page with Tiles
How to define multiple tiles files in struts 2 application?
To define multiple tiles, you need to add following entry in your web.xml file.
<context-param id="struts_tiles"> <param-name>org.apache.tiles.impl.BasicTilesContainer.DEFINITIONS_CONFIG </param-name> <param-value> /WEB-INF/tiles1.xml,/WEB-INF/tiles2.xml</param-value> </context-param>
![]() |
|
Read More