Autocomplete in Struts 2 using Jquery
I have already written a detailed post on Autocompleter Textbox & dropdown in Struts 2 using struts2-dojo-plugin.jar. In this post, I am going to describe how to implement Ajax based autocomplete in Struts 2 web application using jQuery plugin. jQuery Autcomplete is part of the jQuery UI library which allows converting a normal textbox into an autocompleter textbox by providing a data source for the autocompleter values.
Here when user types a character in text box, jQuery will fire an ajax request using autocomplete plugin to Struts 2 action class, which in turn call the dao class which connects to the database and returns the required data back as an array list, this list should be returned in json format to the success function of ajax call. So to handle this you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute (which has getter and setter) into a JSON object. This guide will teach you on how to implementation AJAX in Struts 2 using JQuery and JSON
Library
struts2-json-plugin-2.x.x.jar
ojdbc14.jar
jquery-1.10.2.js
jquery-ui.js
jquery-ui.css
Now create a dynamic web project in eclipse and add the above jars in classpath of the project and the project structure should look like shown below.
Project Structure
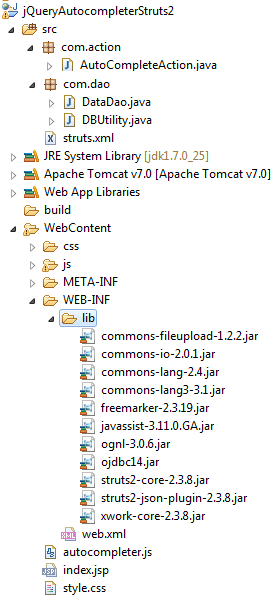
Jsp page
We are done with the setup. Now create a new jsp file under WebContent folder to display the data fetched from database into autocomplete textbox. Now to implement this page with Autocompleter feature and make sure that you referred the jQuery core and jQueryUI libraries.
<%@ taglib prefix="s" uri="/struts-tags"%>Autocomplete in Struts 2 using Jquery and JSON Autocomplete in Struts 2 using Jquery and JSON
Js file
Here we get data from database via ajax and apply autocompleter
$(document).ready(function() { $(function() { $("#search").autocomplete({ source : function(request, response) { $.ajax({ url : "searchAction", type : "POST", data : { term : request.term }, dataType : "json", success : function(jsonResponse) { response(jsonResponse.list); } }); } }); }); });
When a user types a character in text box ,jQuery will fire an ajax request to the controller, in this case controller is SearchController as mentioned in the above js file.
Recommended reading :
Business class
Next step is to create a class that would fetch data from database.
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; public class DataDao { private Connection connection; public DataDao() throws Exception { connection = DBUtility.getConnection(); } public ArrayListgetFrameWork(String frameWork) { ArrayList list = new ArrayList (); PreparedStatement ps = null; String data; try { ps = connection .prepareStatement("SELECT * FROM JAVA_FRAMEWORK WHERE FRAMEWORK LIKE ?"); ps.setString(1, frameWork + "%"); ResultSet rs = ps.executeQuery(); while (rs.next()) { data = rs.getString("FRAMEWORK"); list.add(data); } } catch (Exception e) { System.out.println(e.getMessage()); } return list; } }
Data Access object
Connecting To Database Using JDBC
package com.dao; import java.sql.Connection; import java.sql.DriverManager; public class DBUtility { private static Connection connection = null; public static Connection getConnection() { if (connection != null) return connection; else { // database URL String dbUrl = "jdbc:oracle:thin:@localhost:1521:XE"; try { Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the database connection = DriverManager.getConnection(dbUrl, "system", "admin"); } catch (Exception e) { e.printStackTrace(); } return connection; } } }
Action class
Now Create the action class to handle Ajax call; in action class you need to create instance variables and its respective getter and setter methods since all the variables which have a setter can be set to the values as which are passed as parameters by jQuery and all the variables that have a getter method can be retrieved in the client javascript code.
package com.action; import java.util.ArrayList; import com.dao.DataDao; import com.opensymphony.xwork2.Action; public class AutoCompleteAction implements Action { // Received via Ajax request private String term; // Returned as responce private ArrayListlist; public String execute() { try { System.out.println("Parameter from ajax request : - " + term); DataDao dataDao = new DataDao(); list = dataDao.getFrameWork(term); } catch (Exception e) { System.err.println(e.getMessage()); } return SUCCESS; } public ArrayList getList() { return list; } public void setList(ArrayList list) { this.list = list; } public String getTerm() { return term; } public void setTerm(String term) { this.term = term; } }
As mentioned in the code, the action class will call the business service class which in turn creates the necessary connection and returns the data back as an array list.
Also read :
struts.xml
In struts.xml, create a package that extend json-default and specify the result type of your action class inside this package to be json. This package component is present in struts2-json-plugin-2.x.x.jar
Please read the article on AJAX implementation in Struts 2 using JQuery and JSON to understand about “json-default” package better.
true UTF-8 true
web.xml
Make sure you have done mapping in web.xml file as given below,
struts2 org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter struts2 /* index.jsp
Demo
If you have any other suggestion on above topic, do let me know via comments.
In Action class instead of list assume that you have one object how to populate a textbox
Hai i am getting communication failuer can you please solve me
is your database is “O.K.” to go as Dao may not be getting any value as the database was not… Hope that was a solve for the problem :)
THANK YOU SO MUCH
Most welcome :)
hi i am not able to get value on jsp page though the ui is shown when it matches the text with database but the list in not populated.
Many Thanks to you from me!You helped me a lot!I sucessfully integrate autocomplete!
Most welcome :)
Hi…Your war file working with my database file in mysql . my project integrate your codes but can’t call autocomplter.js file in my project….. please give solutions….