Accessing Map and List attributes in spEL
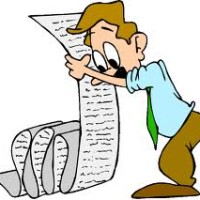
![]() |
|
Step 1 :Create the POJO
The bean is defined using @Component annotation. The values of the Map and List are set in the constructor.
File : Bean.java
package com.simpleCodeStuffs.spEL; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import org.springframework.stereotype.Component; @Component("testBean") public class Bean { private Mapname; private List price; public Bean() { name = new HashMap (); name.put("a", "Sandy"); name.put("b", "Poorni"); name.put("c", "Gokul"); price = new ArrayList (); price.add(150); price.add(200); price.add(570); } public Map getName() { return name; } public void setName(Map name) { this.name = name; } public List getPrice() { return price; } public void setPrice(List price) { this.price = price; } }
Notice how values are injected using spEL in the below class
File : Customer.java
package com.simpleCodeStuffs.spEL; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component("custBean") public class Customer { @Value("#{testBean.name['c']}") private String name; @Value("#{testBean.price[0]}") private double bill; public String getName() { return name; } public void setName(String name) { this.name = name; } public double getBill() { return bill; } public void setBill(double bill) { this.bill = bill; } }
In case of MAP {#beanID.<propertyName>[âMapIndexâ]} In case of List {#beanID.<propertyName>[indexValue]}
Step 2 : Create the main class
File : MainClass.java
package com.simpleCodeStuffs.spEL; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("elBeans.xml"); Customer customer = (Customer) context.getBean("cust"); System.out.println("Customer name: " + customer.getName()); System.out.println("Bill price : " + customer.getBill()); } }
Step 3 :
Note that the values are accessed using annotations in this example. So, do not provide values in the xml as well, as the values provided in xml usually override the values provided through annotations.
File : elBeans.xml
Step 4 : Run the program. The output is

![]() |
|