Setter injection in Spring – example
Dependency injection in this form, injects the values to attributes inside a class through the setter methods present inside the class. A simple POJO(Plain Old Java Object) has only a set of attributes and the corresponding getters and setters. It is a unit at its simplest which does not implement any other special features. Such POJOs are made abstract parts and the dependency is avoided by setting the values from the underlying Spring framework instead of making the values depend on other units/itself.
** UPDATE: Spring Complete tutorial now available here.
1. Create the POJO class into which values are to be injected using setter injection
File : SimpleSetterInjection
package com.simpleCodeStuffs; public class SimpleSetterInjection { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } }
2. Define the metadata
The value for the attribute of the POJO is provided as <property name=” ” value=” ” /> thereby setting the values of these attributes through the setter method.
File : Beans.xml
Step 3:
Write the main class to place a call to this POJO. The object(POJO) is retrieved by passing a call with getBeans(“beanId”) on the context.
File : MainClass.java
package com.simpleCodeStuffs; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); SimpleSetterInjection obj = (SimpleSetterInjection) context.getBean("simpleSetterInjection"); obj.getMessage(); } }
4. Run it
The output appears as

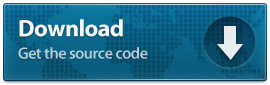