Basic example using spEL
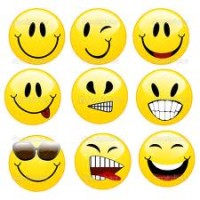
![]() |
|
Step 1 :
Create a new package, com.simpleCodeStuffs.spEL. Create the POJO classes Candidate.java and Address.java with the below specifications.
File : Candidate.java
package com.simpleCodeStuffs.spEL; public class Candidate { private String name; private int age; private Address addrs; private String area; public String getArea() { return area; } public void setArea(String area) { this.area = area; } public Candidate() { this.name = null; this.age = 0; this.addrs = null; } public Candidate(String name, int age, Address addrs) { this.name = name; this.age = age; this.addrs = addrs; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public Address getAddrs() { return addrs; } public void setAddrs(Address addrs) { this.addrs = addrs; } }
Fie : Address.java
package com.simpleCodeStuffs.spEL; public class Address { private String doorNo; private String street; private String area; public Address() { this.doorNo = null; this.street = null; this.area = null; } public Address(String doorNo, String street, String area) { this.doorNo = doorNo; this.street = street; this.area = area; } public String getArea() { return area; } public void setArea(String area) { this.area = area; } public String getDoorNo() { return doorNo; } public void setDoorNo(String doorNo) { this.doorNo = doorNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } }
Step 2 :
Under the src folder, create a new xml file elBeans.xml to provide the configuration metadata using spEL
File ; elBeans.xml
Note here, the value for the bean elAddress is provided using a simple setter injection. But for the bean elCandidate , the value for addrs and area property is provided using spEL expression.
The general syntax for a spEL expression is
#{spEL Expression}
In the above example, the value for addrs is provided as #{elAddress}. This means that addrs is assigned the value set for the bean elAddress. Similarly, for area property, the value is assigned to #{elAddress.area}, thereby injecting the value of the ‘area’ property from ’elAddress’ to the area property inside elCandidate.
Step 3 : Provide the Main Class
File : MainClass.java
package com.simpleCodeStuffs.spEL; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("elBeans.xml"); Candidate can = (Candidate) context.getBean("elCandidate"); Address add = (Address) context.getBean("elAddress"); System.out.println("Name: " + can.getName()); System.out.println("Age : " + can.getAge()); System.out.println("Area : " + can.getArea()); System.out.println("Address : " + can.getAddrs().getDoorNo()); System.out.println("\t " + add.getStreet()); System.out.println("\t " + add.getArea()); } }
Step 4: Run the program. The output is
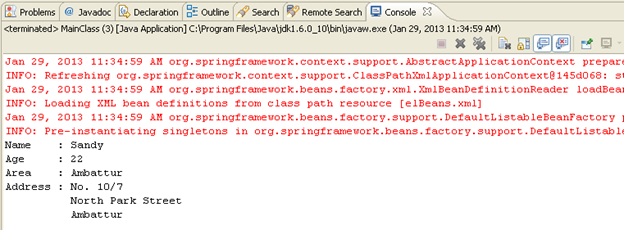
![]() |
|