Bean of Prototype scope
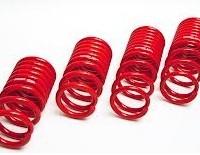
![]() |
|
This scope of bean can be utilised when more than one instance of a particular bean is required during the span of an application. It teturn a new bean instance each time a request is made.
Step 1 : Define the POJO
File : Amount.java
package com.simpleCodeStuffs; public class Amount { private String val; public String getVal() { return val; } public void setVal(String val) { this.val = val; } }
In prototype scope,a new instance of the bean is returned per request. (every time getBean() method is called)
Step 2 : Define the bean definition
File : Beans.xml
Note, only the bean is defined. Property values are injected from the main class in order to provide some clarity on the subject. Also note, the scope has been mentioned as ‘prototype’ in the bean definition. Therefore, an instance of the bean will be returned every time a request is made.
Step 3 : Create the main class
File : MainClass.java
package com.simpleCodeStuffs; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.simpleCodeStuffs.Amount; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); Amount Firstamt=(Amount)context.getBean("amount"); /* instance of bean returned*/ Firstamt.setVal("SimpleCodeStuffs"); System.out.println(Firstamt.getVal()); Amount Secondamt=(Amount)context.getBean("amount"); /*For PROTOTYPE scope, new bean instance is returned */ System.out.println(Secondamt.getVal()); } }
Note that the bean is of scope “prototype”. So an instance of the bean is returned everytime a request is made using getBean() method. So, a new instance is returned for Secondamt and no value has been set for the new instance. This can be seen in output as Secondamt prints null value.
Step 4 : Run the application. The output is
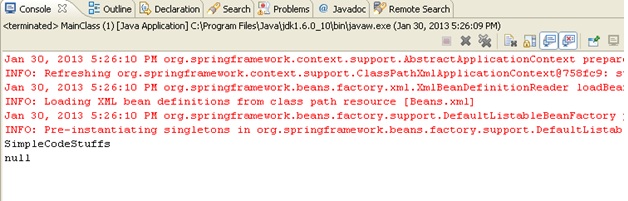
![]() |
|