List FactoryBean, Set FactoryBean and Map FactoryBean in Spring
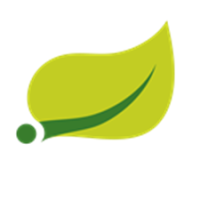
We have previously seen how to deal with List, Set and Map type attributes inside a bean class. Further let us venture into having concrete List collection (ArrayList and LinkedList), Set collection(HashSet and TreeSet)Â Map collection(HashMap and TreeMap)
Step 1 : Create the POJO
File : ClassABC.java
package com.simpleCodeStuffs; import java.util.List; import java.util.Map; import java.util.Set; public class ClassABC { private List listVariable; private Map mapVariable; private Set setVariable; public List getListVariable() { return listVariable; } public void setListVariable(List listVariable) { this.listVariable = listVariable; } public Map getMapVariable() { return mapVariable; } public void setMapVariable(Map mapVariable) { this.mapVariable = mapVariable; } public Set getSetVariable() { return setVariable; } public void setSetVariable(Set setVariable) { this.setVariable = setVariable; } public String toString() { return ("listVariable \t" + listVariable + "\nsetVariable \t" + setVariable + "\nmapVariable \t" + mapVariable); } }
File : Amount.java
package com.simpleCodeStuffs; public class Amount { private String val; public String getVal() { return val; } public void setVal(String val) { this.val = val; } public String toString() { return ("amount bean val: " + val); } }
Step 2 : Provide the configuration metadata in the xml file
To support the concrete classes of List, Map and Set, it is essential to include util schema in the Beans.xml file.Else, you will end up getting a
SAXParseException because of it
File : Beans.xml
2 HashSet
Step 3 :
File : MainClass.java
package com.simpleCodeStuffs; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml"); ClassABC abc = (ClassABC) context.getBean("abc"); System.out.println(abc); } }
Step 4: Run the application. The output is
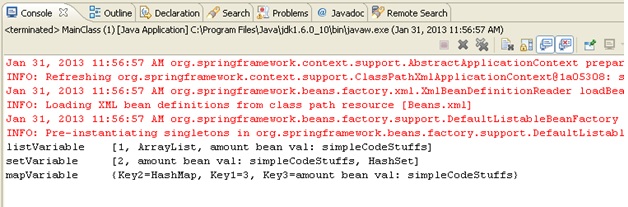