Loading multiple configuration metadata files
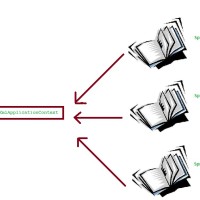
![]() |
|
While dealing with large project structure, more often the bean definitions are segregated and kept in different xml files to maintain the logical/component based structure. In such cases, there is a need to load multiple xml files into the ClassPathXmlApplicationContext.
Step 1 : Consider 3 POJOs, situated in different packages.
Beans.xml has the bean definition of Amount.java
elBeans.xml has the bean definition of Address.java and Candidate.java
File : Amount.java
package com.simpleCodeStuffs; public class Amount { private String val; public String getVal() { return val; } public void setVal(String val) { this.val = val; } public String toString(){ return ("amount bean val: "+val); } }
File : Address.java
package com.simpleCodeStuffs.spEL; public class Address { private String doorNo; private String street; private String area; public Address(){ this.doorNo=null; this.street=null; this.area=null; } public Address(String doorNo, String street,String area){ this.doorNo=doorNo; this.street=street; this.area=area; } public String getArea() { return area; } public void setArea(String area) { this.area = area; } public String getDoorNo() { return doorNo; } public void setDoorNo(String doorNo) { this.doorNo = doorNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String toString(){ return ("\tdoorno "+doorNo+"\n\tStreet "+street+"\n\tarea "+area); } }
File : Candidate.java
package com.simpleCodeStuffs.spEL; import com.simpleCodeStuffs.Amount; public class Candidate { private String name; private int age; private Address addrs; private String area; private Amount amt; public Amount getAmt() { return amt; } public void setAmt(Amount amt) { this.amt = amt; } public String getArea() { return area; } public void setArea(String area) { this.area = area; } public Candidate(){ this.name=null; this.age=0; this.addrs=null; } public Candidate(String name,int age,Address addrs){ this.name=name; this.age=age; this.addrs=addrs; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public Address getAddrs() { return addrs; } public void setAddrs(Address addrs) { this.addrs = addrs; } public String toString(){ return("name" +name+ "age"+age"+"addrs "+addrs"+" amt"+amt); } }
Step 2 :
Notice the package and class structure. Candidate has two other class attributes of which Address is present in the same package and the definition for the corresponding bean is present in the same xml file(elBeans.xml). The class Amount is another attribute inside Candidate, but the class is present in a different package and mainly the bean definition is available in a different xml file(Beans.xml)
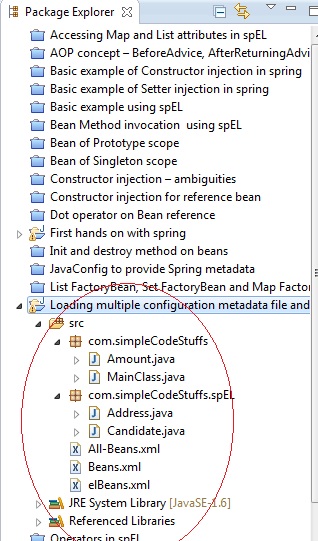
Also, the definition for these beans are provided in different x
Step 3 :
The bean definition for Amount is present in Beans.xml and that for Address and Candidate is present in elBeans.xml
File : Beans.xml
File : elBeans.xml
Step 4: Main class
package com.simpleCodeStuffs; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.simpleCodeStuffs.spEL.Candidate; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext(new String[] {"Beans.xml", "elBeans.xml"}); Candidate can=(Candidate)context.getBean("candidate"); System.out.println(can); } }
The required bean definitions for this application are scattered over two metadata files (Beans.xml and elBeans.xml). Inorder to load both these files, take up the above approach
Step 5 : Run it – Output
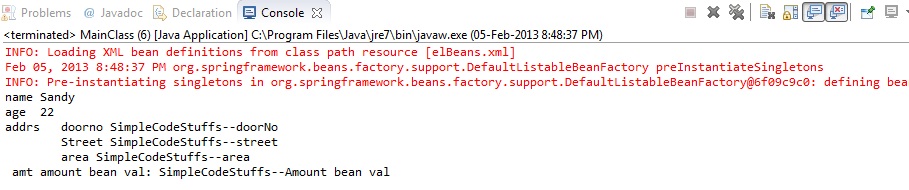
Step 6 : Alternative approach
In case of multiple metadata files, it is always better programming approach to import the various xml files into one xml file and in turn, load this one xml file into the ClassPathXmlApplicationContext
To have a more standard approach towards development, it is better to have a single xml file to cater to all the bean definitions required. Hence, it is advisable to import all the other xml files into one combined xml file and in turn load the one xml file in our ClassPathXmlApplicationContext as a single xml file.
For this, define a new xml file under the src folder, All-Beans.xml
In case the other 2 xmls are present inside different folder structure, then mention as,
.xml/>
Now, load the one bean which has all other metadata imported into it in the main class.
ApplicationContext context = new ClassPathXmlApplicationContext("All-Beans.xml");
The output remains the same. But this programming approach is much better.
![]() |
|