Simple spEL using Annotation
![]() |
|
Just like JavaConfig being an alternate way to provide configuration metadata instead of xml files, Annotations can be used in place of xml metadata in spEL as well.
Step 1 : Create the POJOs, Candidate.java and Address.java
File : Candidate.java
package com.simpleCodeStuffs.spEL; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component("elCandidate") public class Candidate { @Value("Poorni") private String name; @Value("27") private int age; @Value("#{elAddress}") private Address addrs; @Value("#{elAddress.area}") private String area; public String getArea() { return area; } public void setArea(String area) { this.area = area; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public Address getAddrs() { return addrs; } public void setAddrs(Address addrs) { this.addrs = addrs; } }
File : Address.java
package com.simpleCodeStuffs.spEL; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component("elAddress") public class Address { @Value("22/7") private String doorNo; @Value("MKM Street") private String street; @Value("Ambattur") private String area; public String getArea() { return area; } public void setArea(String area) { this.area = area; } public String getDoorNo() { return doorNo; } public void setDoorNo(String doorNo) { this.doorNo = doorNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } }
Step 2 : Enable auto component scanning in the xml file
File : elBeans.xml
Step 3 : Main class
File : MainClass.java
package com.simpleCodeStuffs.spEL; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("elBeans.xml"); Candidate can = (Candidate) context.getBean("elCandidate"); Address add = (Address) context.getBean("elAddress"); System.out.println("Name : " + can.getName()); System.out.println("Age : " + can.getAge()); System.out.println("Area : " + can.getArea()); System.out.println("Address : " + can.getAddrs().getDoorNo()); System.out.println("\t " + add.getStreet()); System.out.println("\t " + add.getArea()); } }
Step 4 :
Run the MainClass. The output is as follows
spelAnnotationOutput
Note that these are the values injected into the bean, through annotation.
Step 5 :
Make modification in the elBeans.xml
Values injected from xml overrides the values from annotation
The bean ‘elCandidate’ is defined. The value for the property ‘age’ is left out here.
File : elBeans.xml
Now, run the program again. The output is
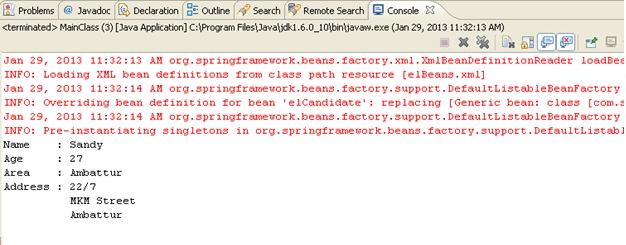
Note that, the value for the attribute ‘name’ provided through xml(Sandy) overrides the one provided through annotation(Poorni). The other values are taken from annotation as they have not been injected from xml.
![]() |
|