Spring AOP – AfterThrows Advice
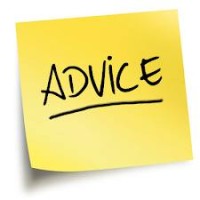
![]() |
|
Requirement : In case a particular method call throws an exception, a log needs to be printed.
** UPDATE: Spring Complete tutorial now available here.
Step 1 :
Changes in IteratorImpl class to mock an exception being thrown. No change in IteratorImpl interface
File : Iterator.java
package com.simpleCodeStuffs.aop; public interface Iterator { void goPrevious(); void goNext(); }
File : IteratorImpl.java
package com.simpleCodeStuffs.aop; public class IteratorImpl implements Iterator{ String flowName; public void goNext() { System.out.println("goNext Method Called on flow - "+flowName); throw new RuntimeException(); } public void goPrevious(){ System.out.println("goPrevious Method called on flow - "+flowName); } public String getFlowName() { return flowName; } public void setFlowName(String flowName) { this.flowName = flowName; } }
Step 2 :
Create a new class AopExampleAfterThrows to implement the new functionality
File : AopExampleAfterThrows.java
package com.simpleCodeStuffs.aop; import org.springframework.aop.ThrowsAdvice; public class AopExampleAfterThrows implements ThrowsAdvice { public void afterThrowing(RuntimeException runtimeException) { System.out.println("Logging step : Exception thrown by the method "); } }
Step 3 :
Changes in the aopBeans.xml file. Similar to the Before Advice and After returns Advice, create the advisor and advice beans
File : aopBeans.xml
com.simpleCodeStuffs.aop.Iterator
theAfterThrowingAdvisor .*
Step 4 :
No major change in the Main class. Simply place a call to the method that throws a mock exception.
File : SpringAopMain.java
package com.simpleCodeStuffs.aop; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.context.support.FileSystemXmlApplicationContext; public class SpringAopMain { public static void main(String[] args) { // Read the configuration file ApplicationContext context = new ClassPathXmlApplicationContext("aopBeans.xml"); // Instantiate an object Iterator IteratorInterface = (Iterator) context .getBean("businesslogicbean"); // Execute the public method of the bean IteratorInterface.goNext(); } }
Step 5 :
Run the Main class. The output is as follows
Please note that the exception shown in the above output is to show the working of the AfterThrows Advice once an exception is thrown. Handling this exception is simple enough. Read on to the next tutorial, wherein in aroundAdvice, this exception has been handled properly.
![]() |
|