Spring AOP – Around Advice
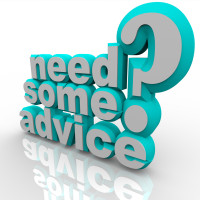
![]() |
|
This can be mentioned as a collation of the before mentioned advices. It does functionality of before, after and throws.
** UPDATE: Spring Complete tutorial now available here.
Step 1 :
No major changes in the Iterator and IteratorImpl classes.
File : Iterator.java
package com.simpleCodeStuffs.aop; public interface Iterator { void goPrevious(); void goNext(); }
File : IteratorImpl.java
package com.simpleCodeStuffs.aop; public class IteratorImpl implements Iterator{ String flowName; public void goNext() { System.out.println("goNext Method Called on flow - "+flowName); } public void goPrevious(){ System.out.println("goPrevious Method called on flow - "+flowName); } public String getFlowName() { return flowName; } public void setFlowName(String flowName) { this.flowName = flowName; } }
Step 2 :
Create a new class AopExampleAroundAdvice
File : AopExampleAroundAdvice.java
package com.simpleCodeStuffs.aop; import org.aopalliance.intercept.MethodInterceptor; import org.aopalliance.intercept.MethodInvocation; public class AopExampleAroundAdvice implements MethodInterceptor{ @Override public Object invoke(MethodInvocation method) throws Throwable { System.out.println("Before invocation of method : "+method.getMethod()); method.proceed(); System.out.println("After execution of method : "+method.getMethod()); return null; } }
Step 3 :
Changes in the aopBeans.xml. Similar to previous tutorials,
- change the value in the list of interceptorNames
- Define the advisor (advice and pattern)
- Define the advice (class reference)
File : aopBeans.xml
com.simpleCodeStuffs.aop.Iterator
theAroundAdvisor .*
Step 4 :
No major change in the Main class. Simply place a call to the methods
File : SpringAopMain.java
package com.simpleCodeStuffs.aop; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class SpringAopMain { public static void main(String[] args) { // Read the configuration file ApplicationContext context = new ClassPathXmlApplicationContext("aopBeans.xml"); // Instantiate an object Iterator IteratorInterface = (Iterator) context.getBean("businesslogicbean"); // Execute the public method of the bean IteratorInterface.goNext(); IteratorInterface.goPrevious(); } }
Step 5 :
Run the main method. The output is as follows
Step 6 :
Lets do a simple modification and check how AroundAdvice works with exceptions thrown.
Insert an exception in the goNext() method of IteratorImpl class to check the working. No change is required in Iterator interface
File : Iterator.java
package com.simpleCodeStuffs.aop; public class IteratorImpl implements Iterator{ String flowName; public void goNext() { System.out.println("goNext Method Called on flow - "+flowName); throw new RuntimeException(); } public void goPrevious(){ System.out.println("goPrevious Method called on flow - "+flowName); } public String getFlowName() { return flowName; } public void setFlowName(String flowName) { this.flowName = flowName; } }
Simply adding a try-catch block in AopExampleAroundAdvice class to handle the exception,
File : AopExampleAroundAdvice.java
package com.simpleCodeStuffs.aop; import org.aopalliance.intercept.MethodInterceptor; import org.aopalliance.intercept.MethodInvocation; public class AopExampleAroundAdvice implements MethodInterceptor{ @Override public Object invoke(MethodInvocation method) throws Throwable { System.out.println("Before invocation of method : "+method.getMethod()); try{ method.proceed(); } catch(RuntimeException e){ System.out.println("Exception -"+e +" caught on method "+method.getMethod()); } System.out.println("After execution of method : "+method.getMethod()); return null; } }
No other change required. The output is as follows:
![]() |
|