ModelDriven Interceptors in Struts 2
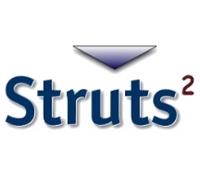
In our last article we have learned how to use a JavaBean class as a property in struts 2 action.
In this tutorial we will learn about Struts 2 support for model objects.
The Model object is a simple JavaBean object, which will be available to action class directly from ValueStack,
i.e we dont need to do deeper referencing to refer its attribute(JavaBeans attribute).
To make a JavaBean object as a model object, our Action class should implement ModelDriven interface which forces the getModel() method to be implemented. This method provides the model object to be pushed into the Value Stack.
Here the getModel() must be invoked earlier for populating its input properties with data from request parameters,
To make sure that the model is available in Value Stack when input parameters are being set, the ModelDrivenInterceptor is configured for the Action in struts.xml.
Most Popular Article for Reference
Since the ModelDriven interceptor is already included in the default stack, we don’t need to inculde modelDriven interceptor explicitly.
** UPDATE: Struts 2 Complete tutorial now available here.
Let’s see a sample example of POJO form, which can be injected in the action as its model.
Bean Class(Model Class)
File : User.java
package com.simplecode.bo; public class User { private String userName; public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } }
Action Class
Action class implements the ModelDriven interface, declared the getModel() method to return the user’s object. When the form data is submitted to this action, it will transfers the form data into the user properties automatically.
File : HelloAction.java
package com.simplecode.action; import com.opensymphony.xwork2.Action; import com.opensymphony.xwork2.ModelDriven; import com.simplecode.bo.User; public class HelloAction implements Action,ModelDriven<User> { // Need to initialize model object private User user = new User(); public String execute() { if (user.getUserName().isEmpty()) return ERROR; else return SUCCESS; } public User getModel() { return user; } }
Note:
1. Since the model object to be pushed into ValueStack must not be null hence model must be initialized before returned by getModel() method.
2. We can use only one model object per action class
Do read :
JSP page
JSP pages for the ModelDriven demonstration.
login.jsp
<%@taglib uri="/struts-tags" prefix="s"%> <html> <head> <title>Login</title> </head> <body> <h3>Struts 2 ModelDriven Example</h3> <s:form action="hello"> <s:textfield name="userName" label="User name"/> <s:submit name="submit" /> </s:form> </body> </html>
success.jsp
<%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>Welcome Page</title> </head> <body> Welcome, <s:property value="userName" /> </body> </html>
error.jsp
<%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>Error Page</title> </head> <body> Login failed! Please enter a valid user name </body> </html>
struts.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" extends="struts-default"> <action name="hello" class="com.simplecode.action.HelloAction"> <result name="success">/jsp/success.jsp</result> <result name="error">/jsp/error.jsp</result> </action> </package> </struts>
Demo
http://localhost:8089/ModelDriven/
When I enter a non empty value in the textbox and press submit button, then the application redirects to success page as shown.
On Clicking submit button without any value in textbox, then the application redirects to error page as shown.