Struts2 AngularJS integration
In this post, we will learn to implement AJAX calls from a JSP page to a Struts 2 Action class using AngularJS and update the same JSP page back with the Json response from the Struts 2.
Library Required
Since Angular can send and receive only in json format, to handle it you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute which has getter and setter into a JSON object.
How to use?
First of all create a “Dynamic Web Project” in Eclipse and download the AngularJS library and place in js folder of eclipse work-space, and refer this files in the head section in the jsp, this file is responsible for the AJAX call made to the Struts2 and for updating the response back in the JSP.
Jsp Page
File: index.jsp
AJAX with Struts 2 using AngularJS First Name : {{person.firstName}}
Last Name : {{person.lastName}}
HTML – Data Binding
We can display JSON data values in jsp by using their attributes in expressions like {{person.firstName}}, {{person.lastName}} etc.. Here the id=”ng-app” is most important for Internet Explorer browser data binding.
ng-controller
Specifies a JavaScript controller class that evaluates HTML expressions.
ng-click
Angular on click event
Model class
package com.model; public class PersonData { private String firstName; private String lastName; // Create Getters and Setters }
Recommended Reading:
- AJAX implementation in Struts 2 using JQuery and JSON
- jQuery Tutorial for Beginners
- GridView in Struts2 using jQuery DataTable via Ajax
Action Class
In action class you need to create instance variables and its respective getter and setter methods. Here all the variables that have a setter can be set to the values received as parameters from AngularJs and all the variables that have a getter method can be retrieved in the AngularJs code.
package com.action; import com.model.PersonData; import com.opensymphony.xwork2.Action; public class AngularAction implements Action { private PersonData personData = new PersonData(); public String execute() { personData.setFirstName("Mohaideen"); personData.setLastName("Jamil"); return SUCCESS; } public PersonData getPersonData() { return personData; } public void setPersonData(PersonData personData) { this.personData = personData; } }
Code Explanation
When the user clicks on “Fetch data from server” button, button click event is fired via angularJS “ng-click” directive and the ‘http’ function executes the Ajax GET request on the Servlet.
struts.xml
Since the response needed by AngularJs is of type json, so we need to convert the PersonData objects to json strings, for this you need to configure Action class in struts.xml such that it returns a json object. This configuration is as follows:
Create a package in struts.xml file, which extend json-default and specify the result type of your action class inside this package to be json. The json-default package contains an interceptor and the result type configuration for JSON requests and responses.
personData true true
Note: json-default package extends struts-default
Web.xml
Struts2 struts2 org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter struts2 /* index.jsp
Recommended Article :
Demo
Once you done with above steps then Right click on project –> Run As –> Run on Server
(Note: I have used tomcat server to run this application. If you are not sure how to configure tomcat server in eclipse please follow this link).
Click button: Fetch data from server to pass HTTP request using angularJS where Java servlet will return data as below:
AngularJS demo
Reference
Read MorePagination in Struts 2 using jQuery jTable plugin
This is the third article on jQuery jTable plugin in Struts 2 that describes on how to implement pagination feature to do server side paging in struts 2 using the JQuery jTable plugin and here I have not explained about how to setup jTable plugin in struts 2. So If you have not read my previous articles “Setting up JQuery jTable plugin in Struts 2” and “Ajax based curd operation in Struts 2 using JQuery jTables plugin”, I will recommend that you read that article first because first one explains how you can integrate the JTable plugin in Struts 2 application and second article explains on how to implement ajax based curd operation. This article will assume that the code for the integration of the jQuery JTable plugin is implemented, and only the code required for implementing pagination in Struts 2 using jTable is explained here.
Setup
As described above, the prerequisite for this code is that you integrate the jQuery jTable plugin into the Struts 2. You can find detailed instructions here, at JQuery jTable plugin in Struts 2, so now I am going to take the sample application I created for explaining AJAX based crud operations in jTable plugin and continue to implement paging for that application.
1. Download sample application from here and import the project in eclipse
2. Follow the steps in this article here to create table in database.
Steps to enable Paging:
From the browser perspective: jTable
To enable paging, paging option must set to true. You can also set pageSize option (default value is 10) in jQuery Script code.
$('#StudentTableContainer').jtable({ //... paging: true, //Set paging enabled pageSize: 3, //Set page size actions: { //... }, fields: { //... } });
Note: pageSize sets the initial number of records to be displayed per page.
Modified Jsp page
jTable Pagination in Java Web Applications jTable Pagination in Java Web Applications
From the server’s perspective: Servlet
If paging is enabled, jTable sends two query string parameters to the server on listAction AJAX call:
• jtStartIndex: Start index of records for current page.
• jtPageSize: Count of maximum expected records.
And it expects additional information from server:
• TotalRecordCount: Total count of records.
In our previous example the url specified in the ‘listAction‘ option has business logic to fetch all records from database. Now in order to handle pagination this ‘listAction’ option should fetch only a part of records for each page, So handle this there are two changes that has to be done in the server side .
1. Modify oracle query to fetch only a subset of records based on the jtStartIndex and jtPageSize.
Since these values are sent along with the request as string parameters by jTable so add following member variable in struts 2 action class and create getters and setters for the same
// Holds Start Page Index private int jtStartIndex; // Hold records to be displayed per Page private int jtPageSize;
2. As mentioned above, jTable need TotalRecordCount to be present in the json response, For which add a member variable totalRecordCount in struts 2 action class and create getters and setters for the same.
Now replace the list method in action class with the below code,
public String list() { try { // Fetch Data from Student Table records = dao.getAllStudents(jtStartIndex, jtPageSize); // Get Total Record Count for Pagination totalRecordCount = dao.getStudentCount(); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); } return Action.SUCCESS; }
Changes made at Dao class
Add below two methods in ‘CrudDao.java’ file
1. Method to get the count of total number of records in the result set.
public int getStudentCount() { int count=0; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery("SELECT COUNT(*) AS COUNT FROM STUDENT"); while (rs.next()) { count=rs.getInt("COUNT"); } } catch (SQLException e) { System.err.println(e.getMessage()); } return count; }
2. In order to return only a subset of records according to the page offset (jtStartIndex and jtPageSize), oracle query should be modified as follows,
In case of Oracle database:
“SELECT * from (Select M.*, Rownum R from STUDENT M) where r > ” + < jtStartIndex> +” and r <= "+< jtStartIndex + jtPageSize >;
In case of MySql database:
select * from STUDENT limit,
Now modify getAllUsers in CurdDao.java using below code
public ListgetAllStudents(int startPageIndex, int recordsPerPage) { List students = new ArrayList (); int range = startPageIndex+recordsPerPage; String query="SELECT * from (Select M.*, Rownum R From STUDENT M) where r >" + startPageIndex +" and r <= "+range; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); while (rs.next()) { Student student = new Student(); student.setStudentId(rs.getInt("STUDENTID")); student.setName(rs.getString("NAME")); student.setDepartment(rs.getString("DEPARTMENT")); student.setEmailId(rs.getString("EMAIL")); students.add(student); } } catch (SQLException e) { System.err.println(e.getMessage()); } return students; }
This function returns only a subset of records according to the page offset (jtStartIndex and jtPageSize).
Now on running the application, with the above changes, the final demo looks as shown below:
Reference
jTable official website
AJAX based CRUD tables using ASP.NET MVC 3 and jTable jQuery plug-in
AJAX implementation in Struts 2 using JQuery and JSON
In this post, we will learn to implement AJAX calls from a JSP page to a Struts 2 Action class using JQuery and update the same JSP page back with the Json response from the Struts 2.
Library required
Since the response to be sent to jQuery is of type JSON, to handle it you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute which has getter and setter into a JSON object.
Steps done to set up our action for JSON
From the browser perspective: jQuery
jQuery allows you to issue an ajax request and expects a JSON object as a response.
Jsp Page
Now, let us create a JSP page with two drop down lists, one contains values for countries and the other that is going to be populated with values for states based on the value selected in the first drop down list. This is done without a page refresh, by making AJAX calls to the Struts 2 action on first drop down list change event.
Recommended reading :
<%@ taglib prefix="s" uri="/struts-tags"%>AJAX in Struts 2 using JSON and jQuery AJAX calls to Struts 2 using JSON and jQuery
Note that I have referenced jQuery files in the head section of the jsp which is responsible for the AJAX call made to the struts 2 action and for displaying the response back in the JSP.
Whenever the value is selected in the “country” drop down list, its change event is fired and the ‘getJSON’ function executes ajaxAction (first argument of ‘getJSON’) configured in struts.xml. I have explained about the parameters of ‘getJSON’ method in the article here.
From the server’s perspective: Struts 2
In action class you need to create instance variables and its respective getter and setter methods. Here all the variables that have a setter can be set to the values received as parameters from jQuery and all the variables that have a getter method can be retrieved in the client javascript code.
Also read :
Action class
package com.action; import java.util.LinkedHashMap; import java.util.Map; import com.opensymphony.xwork2.Action; public class AjaxJsonAction implements Action{ private MapstateMap = new LinkedHashMap (); private String dummyMsg; //Parameter from Jquery private String countryName; public String execute() { if (countryName.equals("India")) { stateMap.put("1", "Kerala"); stateMap.put("2", "Tamil Nadu"); stateMap.put("3", "Jammu Kashmir"); stateMap.put("4", "Assam"); } else if (countryName.equals("US")) { stateMap.put("1", "Georgia"); stateMap.put("2", "Utah"); stateMap.put("3", "Texas"); stateMap.put("4", "New Jersey"); } else if (countryName.equals("Select Country")) { stateMap.put("1", "Select State"); } dummyMsg = "Ajax action Triggered"; return SUCCESS; } public Map getStateMap() { return stateMap; } public String getDummyMsg() { return dummyMsg; } public String getCountryName() { return countryName; } public void setStateMap(Map stateMap) { this.stateMap = stateMap; } public void setDummyMsg(String dummyMsg) { this.dummyMsg = dummyMsg; } public void setCountryName(String countryName) { this.countryName = countryName; } }
In the above code, we create a maps and populates its value based on the country parameter passed to the action class by the AJAX call made by the JQuery’s getJSON() method.
struts.xml
Since the response needed by jQuery is of type json, so we need to convert this map objects to json strings, for this you need to configure this Action class in struts.xml such that it returns a json object. This configuration is as follows:
Create a package in struts.xml file, which extend json-default and specify the result type of your action class inside this package to be json. The json-default package contains an interceptor and the result type configuration for JSON requests and responses.
true true
Note: json-default package extends struts-default
Web.xml
Struts2 struts2 org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter struts2 /* index.jsp
Recommended Article :
Demo
On selecting ‘India’ in the first dropdown list
On selecting ‘US’ in the first dropdown list
Autocomplete in Struts 2 using Jquery
I have already written a detailed post on Autocompleter Textbox & dropdown in Struts 2 using struts2-dojo-plugin.jar. In this post, I am going to describe how to implement Ajax based autocomplete in Struts 2 web application using jQuery plugin. jQuery Autcomplete is part of the jQuery UI library which allows converting a normal textbox into an autocompleter textbox by providing a data source for the autocompleter values.
Here when user types a character in text box, jQuery will fire an ajax request using autocomplete plugin to Struts 2 action class, which in turn call the dao class which connects to the database and returns the required data back as an array list, this list should be returned in json format to the success function of ajax call. So to handle this you need struts2-json-plugin-2.x.x.jar. This plugin allows you to serialize the Action class attribute (which has getter and setter) into a JSON object. This guide will teach you on how to implementation AJAX in Struts 2 using JQuery and JSON
Library
struts2-json-plugin-2.x.x.jar
ojdbc14.jar
jquery-1.10.2.js
jquery-ui.js
jquery-ui.css
Now create a dynamic web project in eclipse and add the above jars in classpath of the project and the project structure should look like shown below.
Project Structure
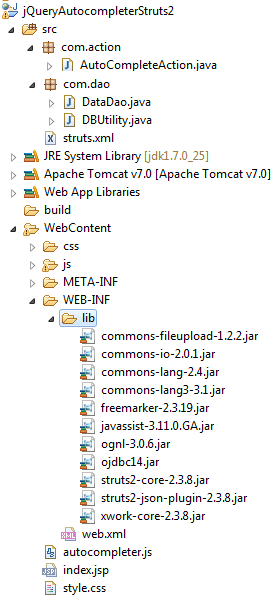
Jsp page
We are done with the setup. Now create a new jsp file under WebContent folder to display the data fetched from database into autocomplete textbox. Now to implement this page with Autocompleter feature and make sure that you referred the jQuery core and jQueryUI libraries.
<%@ taglib prefix="s" uri="/struts-tags"%>Autocomplete in Struts 2 using Jquery and JSON Autocomplete in Struts 2 using Jquery and JSON
Js file
Here we get data from database via ajax and apply autocompleter
$(document).ready(function() { $(function() { $("#search").autocomplete({ source : function(request, response) { $.ajax({ url : "searchAction", type : "POST", data : { term : request.term }, dataType : "json", success : function(jsonResponse) { response(jsonResponse.list); } }); } }); }); });
When a user types a character in text box ,jQuery will fire an ajax request to the controller, in this case controller is SearchController as mentioned in the above js file.
Recommended reading :
Business class
Next step is to create a class that would fetch data from database.
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; public class DataDao { private Connection connection; public DataDao() throws Exception { connection = DBUtility.getConnection(); } public ArrayListgetFrameWork(String frameWork) { ArrayList list = new ArrayList (); PreparedStatement ps = null; String data; try { ps = connection .prepareStatement("SELECT * FROM JAVA_FRAMEWORK WHERE FRAMEWORK LIKE ?"); ps.setString(1, frameWork + "%"); ResultSet rs = ps.executeQuery(); while (rs.next()) { data = rs.getString("FRAMEWORK"); list.add(data); } } catch (Exception e) { System.out.println(e.getMessage()); } return list; } }
Data Access object
Connecting To Database Using JDBC
package com.dao; import java.sql.Connection; import java.sql.DriverManager; public class DBUtility { private static Connection connection = null; public static Connection getConnection() { if (connection != null) return connection; else { // database URL String dbUrl = "jdbc:oracle:thin:@localhost:1521:XE"; try { Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the database connection = DriverManager.getConnection(dbUrl, "system", "admin"); } catch (Exception e) { e.printStackTrace(); } return connection; } } }
Action class
Now Create the action class to handle Ajax call; in action class you need to create instance variables and its respective getter and setter methods since all the variables which have a setter can be set to the values as which are passed as parameters by jQuery and all the variables that have a getter method can be retrieved in the client javascript code.
package com.action; import java.util.ArrayList; import com.dao.DataDao; import com.opensymphony.xwork2.Action; public class AutoCompleteAction implements Action { // Received via Ajax request private String term; // Returned as responce private ArrayListlist; public String execute() { try { System.out.println("Parameter from ajax request : - " + term); DataDao dataDao = new DataDao(); list = dataDao.getFrameWork(term); } catch (Exception e) { System.err.println(e.getMessage()); } return SUCCESS; } public ArrayList getList() { return list; } public void setList(ArrayList list) { this.list = list; } public String getTerm() { return term; } public void setTerm(String term) { this.term = term; } }
As mentioned in the code, the action class will call the business service class which in turn creates the necessary connection and returns the data back as an array list.
Also read :
struts.xml
In struts.xml, create a package that extend json-default and specify the result type of your action class inside this package to be json. This package component is present in struts2-json-plugin-2.x.x.jar
Please read the article on AJAX implementation in Struts 2 using JQuery and JSON to understand about “json-default” package better.
true UTF-8 true
web.xml
Make sure you have done mapping in web.xml file as given below,
struts2 org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter struts2 /* index.jsp
Demo
If you have any other suggestion on above topic, do let me know via comments.
CRUD Operations in Struts 2 using jTable jQuery plugin via Ajax
In the previous article “Setting up jQuery jTable plugin with Struts 2 framework” I have explained about how to setup jTable plugin in struts 2 application. This article describes on how to implement “Ajax based curd operation in Struts 2 using the JQuery jTable plugin, If you have not read the previous articles “Setting up jQuery jTable plugin with Struts 2 framework” I will recommend that you read that article first because it explains what jTable plugin is and how you can integrate it in Struts 2 application.
Steps done to set up our application for jTable
Libraries required for the setup,
- jQuery
- jQuery UI
- jTable
- struts2-json-plugin-2.x.x.jar
- Commonly required Struts 2 jars
Create a dynamic project in eclipse and setup above required libraries as explained here. The final project structure of this looks as below.
Setup from the browser perspective: jTable
JSP
jTable in Struts 2 AJAX based CRUD operation in Struts 2 using jTable plugin
JS file for implementing CRUD
$(document).ready(function() { $('#StudentTableContainer').jtable({ title : 'Students List', actions : { listAction : 'listAction', createAction : 'createAction', updateAction : 'updateAction', deleteAction : 'deleteAction' }, fields : { studentId : { title : 'Student Id', width : '30%', key : true, list : true, edit : false, create : true }, name : { title : 'Name', width : '30%', edit : true }, department : { title : 'Department', width : '30%', edit : true }, emailId : { title : 'Email', width : '20%', edit : true } } }); $('#StudentTableContainer').jtable('load'); });
I have explained the working of above jTable js file in my previous article “Setting up JQuery jTable plugin in Struts 2”, hence I’m not going to explain it again.
Now create a student table in Oracle database using the query below. On this table we are going to perform CRUD operation via ajax
create table Student(studentid int,name varchar(50),department varchar(50), email varchar(50));
CurdDao
Create a class that performs CRUD operation in database
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.List; import com.jdbc.DataAccessObject; import com.model.Student; public class CrudDao { private Connection dbConnection; private PreparedStatement pStmt; public CrudDao() { dbConnection = DataAccessObject.getConnection(); } public void addStudent(Student student) { String insertQuery ="INSERT INTO STUDENT(STUDENTID, NAME," + "DEPARTMENT, EMAIL) VALUES (?,?,?,?)"; try { pStmt = dbConnection.prepareStatement(insertQuery); pStmt.setInt(1, student.getStudentId()); pStmt.setString(2, student.getName()); pStmt.setString(3, student.getDepartment()); pStmt.setString(4, student.getEmailId()); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public void deleteStudent(int userId) { String deleteQuery = "DELETE FROM STUDENT WHERE STUDENTID = ?"; try { pStmt = dbConnection.prepareStatement(deleteQuery); pStmt.setInt(1, userId); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public void updateStudent(Student student) { String updateQuery ="UPDATE STUDENT SET NAME = ?," + "DEPARTMENT = ?, EMAIL = ? WHERE STUDENTID = ?"; try { pStmt = dbConnection.prepareStatement(updateQuery); pStmt.setString(1, student.getName()); pStmt.setString(2, student.getDepartment()); pStmt.setString(3, student.getEmailId()); pStmt.setInt(4, student.getStudentId()); pStmt.executeUpdate(); } catch (SQLException e) { System.err.println(e.getMessage()); } } public ListgetAllStudents() { List students = new ArrayList (); String query = "SELECT * FROM STUDENT ORDER BY STUDENTID"; try { Statement stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); while (rs.next()) { Student student = new Student(); student.setStudentId(rs.getInt("STUDENTID")); student.setName(rs.getString("NAME")); student.setDepartment(rs.getString("DEPARTMENT")); student.setEmailId(rs.getString("EMAIL")); students.add(student); } } catch (SQLException e) { System.err.println(e.getMessage()); } return students; } }
I hope the above code is self explanatory
Setup from the server’s perspective: Servlet
In Struts 2 Action class, I have defined 4 method- create, read, update and delete to perform CRUD operations. Since jTable accepts data only in Json format, so we are converting this List (Java Object) to Json(Javascript object Notation) format using struts2-json-plugin.jar.
**Update: In the article AJAX implementation in Struts 2 using JQuery and JSON I have explained in detail about how to use struts2-json-plugin.jar clearly, So if you are not aware of how struts2-json-plugin works, then please go thorough the above mentioned link.
Action class
package com.action; import java.io.IOException; import java.util.List; import com.dao.CrudDao; import com.model.Student; import com.opensymphony.xwork2.Action; public class JtableAction { private CrudDao dao = new CrudDao(); private Listrecords; private String result; private String message; private Student record; private int studentId; private String name; private String department; private String emailId; public String list() { try { // Fetch Data from Student Table records = dao.getAllStudents(); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String create() throws IOException { record = new Student(); record.setStudentId(studentId); record.setName(name); record.setDepartment(department); record.setEmailId(emailId); try { // Create new record dao.addStudent(record); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String update() throws IOException { Student student = new Student(); student.setStudentId(studentId); student.setName(name); student.setDepartment(department); student.setEmailId(emailId); try { // Update existing record dao.updateStudent(student); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public String delete() throws IOException { // Delete record try { dao.deleteStudent(studentId); result = "OK"; } catch (Exception e) { result = "ERROR"; message = e.getMessage(); System.err.println(e.getMessage()); } return Action.SUCCESS; } public int getStudentId() { return studentId; } public String getName() { return name; } public String getDepartment() { return department; } public String getEmailId() { return emailId; } public void setStudentId(int studentId) { this.studentId = studentId; } public void setName(String name) { this.name = name; } public void setDepartment(String department) { this.department = department; } public void setEmailId(String emailId) { this.emailId = emailId; } public Student getRecord() { return record; } public void setRecord(Student record) { this.record = record; } public List getRecords() { return records; } public String getResult() { return result; } public String getMessage() { return message; } public void setRecords(List records) { this.records = records; } public void setResult(String result) { this.result = result; } public void setMessage(String message) { this.message = message; } }
If you read my article on CRUD Operations in Java Web Applications using jTable jQuery plugin via Ajax then you might have noted once difference here, i.e. I have not created any request or response object in action class to get the student parameters, because those parameter from jsp file auto bounded to my struts 2 action, this is done via struts2-jquery-plugin. One only requirement for this parameter to be passed from jsp is, you have create the member variable for those parameter in action class along with getters and setters as in above file.
You might be interested to read:
I have explained in detail about difference response generated for create, read, update and delete operation in the article CRUD Operations in Java Web Applications using jTable jQuery plugin via Ajax, So please refer to this article mentioned above, if you are not aware of the different response created for CRUD operation in Jtable plugin.
Jtable Issue related to Struts 2
As mentioned in my previous article , the property names of jTable plugin are case sensitive. Only “Result”, “Records” and “Message” will work. In struts 2 the “json response” generated is in lower case[“result”, “records” and “message”], hence I edited the jtable.js to replace Result to result, Records to records and Message to message then it worked.
**Note: Along with the above keyword replace TotalRecordCount to totalRecordCount, since this parameter will be used to display pagination count (Which I will implement in upcoming tutorial)
Model class
Create Model class used in the controller, which will have getters and setters for fields specified in jTable script.
package com.model; public class Student { private int studentId; private String name; private String department; private String emailId; public int getStudentId() { return studentId; } public String getName() { return name; } public String getDepartment() { return department; } public String getEmailId() { return emailId; } public void setStudentId(int studentId) { this.studentId = studentId; } public void setName(String name) { this.name = name; } public void setDepartment(String department) { this.department = department; } public void setEmailId(String emailId) { this.emailId = emailId; } }
DAO Class
Create utility class which connect to database Using Oracle JDBC driver
package com.jdbc; import java.sql.Connection; import java.sql.DriverManager; public class DataAccessObject { private static Connection connection = null; public static Connection getConnection() { if (connection != null) return connection; else { // database URL String dbUrl = "jdbc:oracle:thin:@localhost:1521:XE"; try { Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the database connection = DriverManager.getConnection(dbUrl, "system", "admin"); } catch (Exception e) { e.printStackTrace(); } return connection; } } }
Struts.xml
Make sure that you have the following mapping in struts.xml
/index.jsp
web.xml
struts2 org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter struts2 /* index.jsp
Demo
On running the application
On clicking ‘Add new record’
Now the new record will be added with fade out animation
On clicking edit button
On clicking delete button
In the next article Pagination in Struts 2 using jQuery jTable plugin I have implemented paging feature in the CRUD example.
Reference
jTable official website
AJAX based CRUD tables using ASP.NET MVC 3 and jTable jQuery plug-in
FieldError in Struts 2 Example
In our previous tutorial we learnt about actionError and actionMessage in struts 2, in this tutorial, we are going to describe the fielderror tags. The fielderror tag is a UI tag that renders field errors if they exist.
** UPDATE: Struts 2 Complete tutorial now available here.
Folder Structure
Action Class
Develop an action class using addFieldError(String fieldName, String errorMessage)
method
. This method adds an error message for a given field to the corresponding jsp page.
Here the error messages is added to each field individually using the addFieldError() method. The error messages can either be specified in a separate property file or can be added directly in the Action class itself.
package com.action; import com.opensymphony.xwork2.ActionSupport; public class LoginAction extends ActionSupport { private static final long serialVersionUID = 6677091252031583948L; private String userName; private String password; public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String execute() { return SUCCESS; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public void validate() { if (userName.isEmpty()) { addFieldError("userName", "Username can't be blank"); } if (password.isEmpty()) { addFieldError("password", "Password Can't be blank"); } else { addActionMessage("Welcome " + userName + ", You have been Successfully Logged in"); } } }
Here when either of userName or password is empty then fieldError is added this action via addFiedError method, so the execute method does not get invoked, and interceptors take case altering the flow of response by sending “error” string.
If userName and password are valid then a success message is added to actionMessage, the execute method get invoked, the jsp page get displayed based on execute methods returned value.
Recommended Article
JSP
File: Login.jsp
Create a jsp page that will display your field error messages (when fails to logged-in) using <s:fielderror/>
tag as shown:
<%@taglib uri="/struts-tags" prefix="s"%>Login FieldError in Struts 2
File : Success.jsp
<%@ taglib prefix="s" uri="/struts-tags"%>Welcome Page ActionError & ActionMessage Example
We have done the coding such that If the username or password is empty , then the user will be forwarded to login.jsp where the field error added using addFieldError will get displayed.
You might be interested to read:
struts.xml
Make sure that the struts.xml has the following mapping
success.jsp login.jsp
The web.xml configuration is same as given in hello world programe.
Demo
On running the application
When Username or password is empty, display error message set by <s:fielderror/>
When Username and password is non empty, then application executes successfully and redirects to success.jsp and displays the ActionMessage setted in Validate method via <s:actionmessage/> tag.
Reference
FieldError Apache