Comparison of Performance between different for loops in java
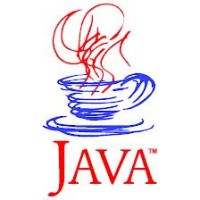
In this post, we will compare the performance of different for loops in java
Different ways to use for loop
1) For each statement
In this technique, advanced for each statement introduced in java 5 is used.
private static List<Integer> list = new ArrayList<>(); for(Integer i : list) { // logic here }
2) Using list.size() in condition
private static List<Integer> list = new ArrayList<>(); for(int j = 0; j < list.size() ; j++) { // logic here }
3) Initialize another local variable with size
private static List<Integer> list = new ArrayList<>(); int size = list.size(); for(int j = 0; j < size ; j++) { // logic here }
4) Initialize the initial value of counter to size of list
private static List<Integer> list = new ArrayList<>(); for(int j = list.size(); j > size ; j--) { // logic here }
Comparing the performance of all types
import java.util.List; import java.util.Calendar; import java.util.ArrayList; public class ForLoopPerformance { private static long startTime; private static long endTime; private static List<Integer> list = new ArrayList<>(); static { for(int i=0; i < 10000000; i++) { list.add(i); } } public static void main(String[] args) { //Type 1 startTime = Calendar.getInstance().getTimeInMillis(); for(Integer i : list) { // logic here } endTime = Calendar.getInstance().getTimeInMillis(); System.out.println("For each loop :: " + (endTime - startTime) + " ms"); //Type 2 startTime = Calendar.getInstance().getTimeInMillis(); for(int j = 0; j < list.size() ; j++) { // logic here } endTime = Calendar.getInstance().getTimeInMillis(); System.out.println("Using collection.size() :: " + (endTime - startTime) + " ms"); //Type 3 startTime = Calendar.getInstance().getTimeInMillis(); int size = list.size(); for(int j = 0; j < size ; j++) { // logic here } endTime = Calendar.getInstance().getTimeInMillis(); System.out.println("Using [int size = list.size(); int j = 0; j < size ; j++] :: " + (endTime - startTime) + " ms"); //Type 4 startTime = Calendar.getInstance().getTimeInMillis(); for(int j = list.size(); j > size ; j--) { // logic here } endTime = Calendar.getInstance().getTimeInMillis(); System.out.println("Using [int j = list.size(); j > size ; j--] :: " + (endTime - startTime) + " ms"); } }
Run it :
Using collection.size() :: 36 ms
Using [int size = list.size(); int j = 0; j < size ; j++] :: 4 ms
Using [int j = list.size(); j > size ; j--] :: 1 ms
Reason for variation in performance
for-each loop(Type 1) is costliest among 4 types, because it uses an Iterator behind the scenes which adds up to most of cost which is not involved in direct access in other three types.
Type 2 uses size() method calls every time and thus on runtime it brings a little overhead.
Type 3 and 4 have a very little difference, they both fetch the size of collection initially. And then uses this size value in loop for checking the condition.