Autocomplete in java web application using Jquery and JSON
This article will describe how to implement jQuery Autocomplete in java web application. jQuery Autcomplete is part of the jQuery UI library which allows converting a normal textbox into an autocompleter textbox by providing a data source for the autocompleter values.
Here when user types a character in text box ,jQuery will fire an ajax request using autocomplete plugin to the controller, this controller(Servlet) in turn call the dao class which connects to the database and returns the required data back as an array list. After getting the data we convert this list to json format and return it back to the success function of our ajax call.
Library
gson-2.2.2.jar
ojdbc14.jar
servlet-api.jar
jquery-1.10.2.js
jquery-ui.js
jquery-ui.css
Project Structure
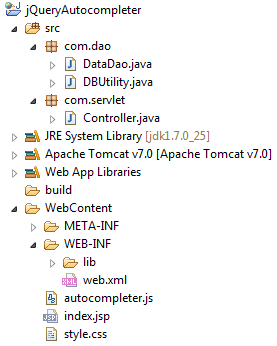
Jsp page
Now create a jsp page with Autocompleter feature and make sure that you referred the jQuery core and jQueryUI libraries.
Autocomplete in java web application using Jquery and JSON Autocomplete in java web application using Jquery and JSON
Recommended reading:
Js file
File: autocompleter.js
In this file we get data from database via ajax and apply autocompleter
$(document).ready(function() { $(function() { $("#search").autocomplete({ source : function(request, response) { $.ajax({ url : "SearchController", type : "GET", data : { term : request.term }, dataType : "json", success : function(data) { response(data); } }); } }); }); });
When a user types a character in text box ,jQuery will fire an ajax request to the controller, in this case controller is SearchController as mentioned in the above js file.
Controller
Creating The Controller To Handle Ajax Calls
package com.servlet; import java.io.IOException; import java.util.ArrayList; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.dao.DataDao; import com.google.gson.Gson; public class Controller extends HttpServlet { private static final long serialVersionUID = 1L; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("application/json"); try { String term = request.getParameter("term"); System.out.println("Data from ajax call " + term); DataDao dataDao = new DataDao(); ArrayListlist = dataDao.getFrameWork(term); String searchList = new Gson().toJson(list); response.getWriter().write(searchList); } catch (Exception e) { System.err.println(e.getMessage()); } } }
This servlet will call the business class which in turn creates the necessary connection and returns the data back as an array list to the controller. After getting the data we convert it to json format and return it back to the success function of our ajax call.
Do read
Business class
Creating Methods To Get Data From Database
package com.dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; public class DataDao { private Connection connection; public DataDao() throws Exception { connection = DBUtility.getConnection(); } public ArrayListgetFrameWork(String frameWork) { ArrayList list = new ArrayList (); PreparedStatement ps = null; String data; try { ps = connection.prepareStatement("SELECT * FROM JAVA_FRAMEWORK WHERE FRAMEWORK LIKE ?"); ps.setString(1, frameWork + "%"); ResultSet rs = ps.executeQuery(); while (rs.next()) { data = rs.getString("FRAMEWORK"); list.add(data); } } catch (Exception e) { System.out.println(e.getMessage()); } return list; } }
Data Access object
Connecting To Database Using JDBC
package com.dao; import java.sql.Connection; import java.sql.DriverManager; public class DBUtility { private static Connection connection = null; public static Connection getConnection() throws Exception { if (connection != null) return connection; else { // Store the database URL in a string String serverName = "127.0.0.1"; String portNumber = "1521"; String sid = "XE"; String dbUrl = "jdbc:oracle:thin:@" + serverName + ":" + portNumber + ":" + sid; Class.forName("oracle.jdbc.driver.OracleDriver"); // set the url, username and password for the databse connection = DriverManager.getConnection(dbUrl, "system", "admin"); return connection; } } }
web.xml
Make sure you have done servlet mapping properly in web.xml file. An example of this is given below,
index.jsp SearchController com.servlet.Controller SearchController /SearchController
Demo
this gets only one register. how i can do with more?
Hey Not gettin
Where to look for autocompleter.js?
I have tried your suggestion to implement autocomplete feature in our web application. But unfortunately, I am getting object expected script error while typing the text in text box
Hi, Above Example is working fine. But we get null error after getting the value in the textbox. why?