RECENT POSTS
Basic example of Setter injection in spring
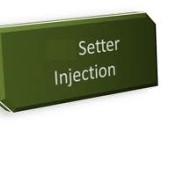
Download It â Basic example of Setter injection in spring Dependency injection in this form, injects the values to attributes inside a class through the setter methods present inside the class. A simple POJO(Plain Old Java Object) has only a set of attributes and the corresponding getters and setters. It is a unit at its simplest which does not implement any other special features. Such POJOs are made abstract parts and the dependency is avoided by setting the values from the underlying Spring framework...
read moreHow To Run A MySQL Script Using Java
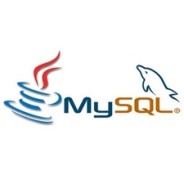
In this tutorial, we shall learn about running a MySQL script file using ibatis ScriptRunner class. Download the following libraries and add them into your classpath. Download It – ibatis & Mysql JDBC Driver package com.simplecode.jdbc; import java.io.Reader; import java.io.FileReader; import java.io.BufferedReader; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import...
read moreFile Upload Example in Struts 2
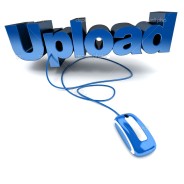
In Struts 2 file uploading can done with the help of built-in FileUploadInterceptor. We use <s:file> tag to create a file upload component, which allow users to select file from their local disk and upload it to the server. The encoding type of the form should be set to multipart/form-data and the HTTP method should be set to post. The Struts 2 File Upload Interceptor is based on MultiPartRequestWrapper, which is automatically applied to the request if it contains the file element. Then it adds the following parameters to the...
read moreRead / Write CSV file in Java using opencsv library
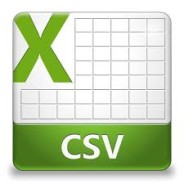
We often need to read data from a CSV file to do some manipulation. Most of the examples I have seen uses StringTokenizer to read the CSV file but that has certain limitations. It cannot read a CSV file properly if the data present in the CSV has a comma in them. We can avoid this issue by using a open source project called Opencsv You can use this library to Create, Read and write CSV files. The best part of OpenCSV parser is, it takes a CSV file and map the result data to a Java Bean object. Please download the...
read moreJava code to Auto size columns in Excel files created with Apache POI
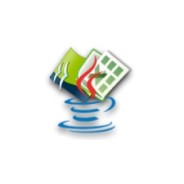
This was the reports created by my java application and it turned out to be quite irritating as the columns are not auto sized. The solution to the above problem is you just need to call a method to auto fit the column width. Here is the code to auto size the first 5 columns of the spread sheet. HSSFSheet sheet = workBook.createSheet("testXls"); // Code to Auto size the column widths for(int columnPosition = 0; columnPosition< 5; columnPosition++) { ...
read moreWhy final variable in for-each loop does not act final?
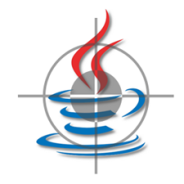
public class ForEachLoop { public static void main(String[] args) { String[] vowels = { "a", "e", "i", "o", "u" }; for (final String str : vowels) { System.out.println(str); } } } Since the String str is declared as final, this code should not compile. However, this is working fine, because internal the structure of for-each loop is actually just as show below: public class ForEachLoop { public static void main(String[] args)...
read moreWhen to use Comparator and Comparable in Java
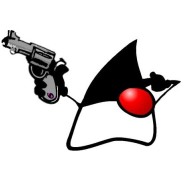
In this post let’s see some best practices and recommendation on when to use Comparator or Comparable in Java: Please consider the below point if you are about to perform sorting. 1) If the object is sorted by single way then it should implement Comparable interface, for example, let’s assume you have a Student bean class which has name, rank and id as member variable. In this case if you are about to perform sorting only by using id then you can go for Comparable interface. On the other hand if an Object can be sorted on multiple...
read more