Struts 2 Push Tag Example
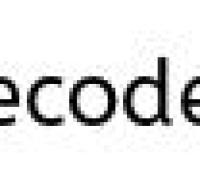
Struts 2 “push” tag is used to push a value into the ValueStack. The value we pushed using push tag will be on top of the ValueStack, so it can be easily referenced using the first-level OGNL expression instead of a deeper reference. The following code show how to do this.
** UPDATE: Struts 2 Complete tutorial now available here.
![]() |
|
1. Action
package com.simplecode.action; import com.simplecode.util.AuthorBean; //PushTag Action class public class BookInfoAction { private AuthorBean authorBean; public String populate() { authorBean = new AuthorBean ("Mohammed masjid", "Akuland Nz","8051 Micro Controller"); return "populate"; } public String execute() { return "success"; } public AuthorBean getAuthorBean() { return authorBean; } public void setAuthorBean(AuthorBean authorBean) { this.authorBean = authorBean; } }
2. Bean
A simple AuthorBean class, later will push it into the stack for easy access.
package com.simplecode.util; public class AuthorBean { private String name; private String university; private String book; public AuthorBean(String name, String university, String book) { this.name = name; this.university = university; this.book = book; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getUniversity() { return university; } public void setUniversity(String university) { this.university = university; } public String getBook() { return book; } public void setBook(String book) { this.book = book; } }
3. Push tag example
It shows the use of “push” tag.
<%@taglib uri="/struts-tags" prefix="s"%> <html> <head> <title>Book Details</title> </head> <body> <p>Author name property can be accessed in two ways:</p> <br /> (Method 1 - Normal method) <br /> <b>Author Name:</b> <s:property value="authorBean.name" /> <br /> <b> University: </b> <s:property value="authorBean.university" /> <br/><b>Book Title :</b> <s:property value="authorBean.book" /> <br /> <br /> (Method 2-push tag) <s:push value="authorBean"> <b>Author Name:</b> <s:property value="name" /> <b> University: </b> <s:property value="university" /> <b>Book Title :</b> <s:property value="book" /> </s:push> </body> </html>
How it work?
Normally, if you want to get the bean’s property, you may reference it like
<s:property value="authorBean.name" />
With “push” tag, you can push the “authorBean” to the top of the stack, and access the property directly
<s:property value="name" />
Both are returned the same result, but with different access mechanism only.
4. Struts.xml
Link it
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" extends="struts-default"> <action name="*BookAction" method="{1}" class="com.simplecode.action.BookInfoAction"> <result name="populate">/bookDetails.jsp</result> </action> </package> </struts>
5. Demo
http://localhost:8089/PushTag/populateBookAction.action
Run it